
Java Tutorials
Introduction to java programming
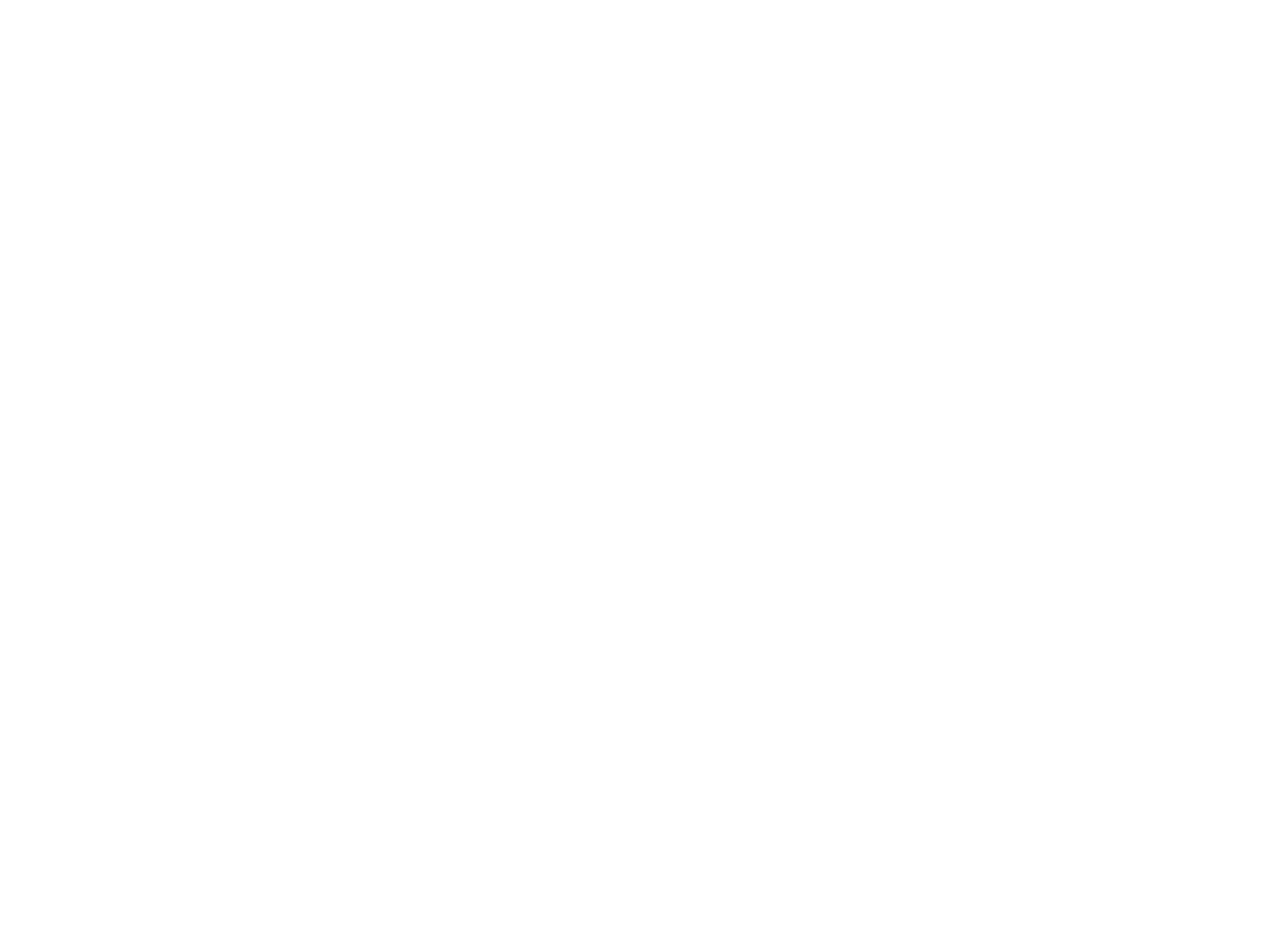
ModernCompilerDesign
JavaTutorial
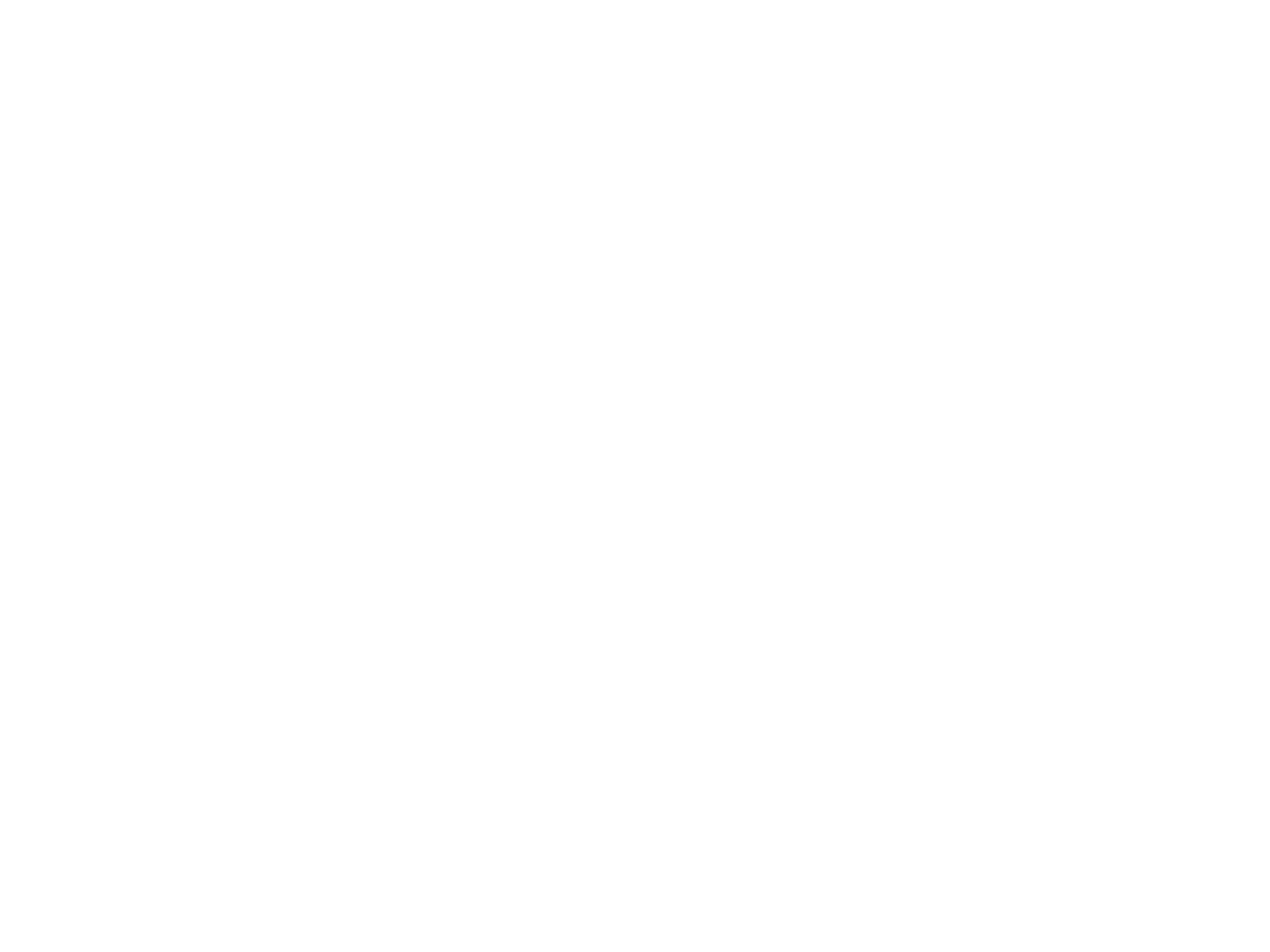
Object-OrientedProgramming
PartlyadaptedwithpermissionfromEranToch,Technion
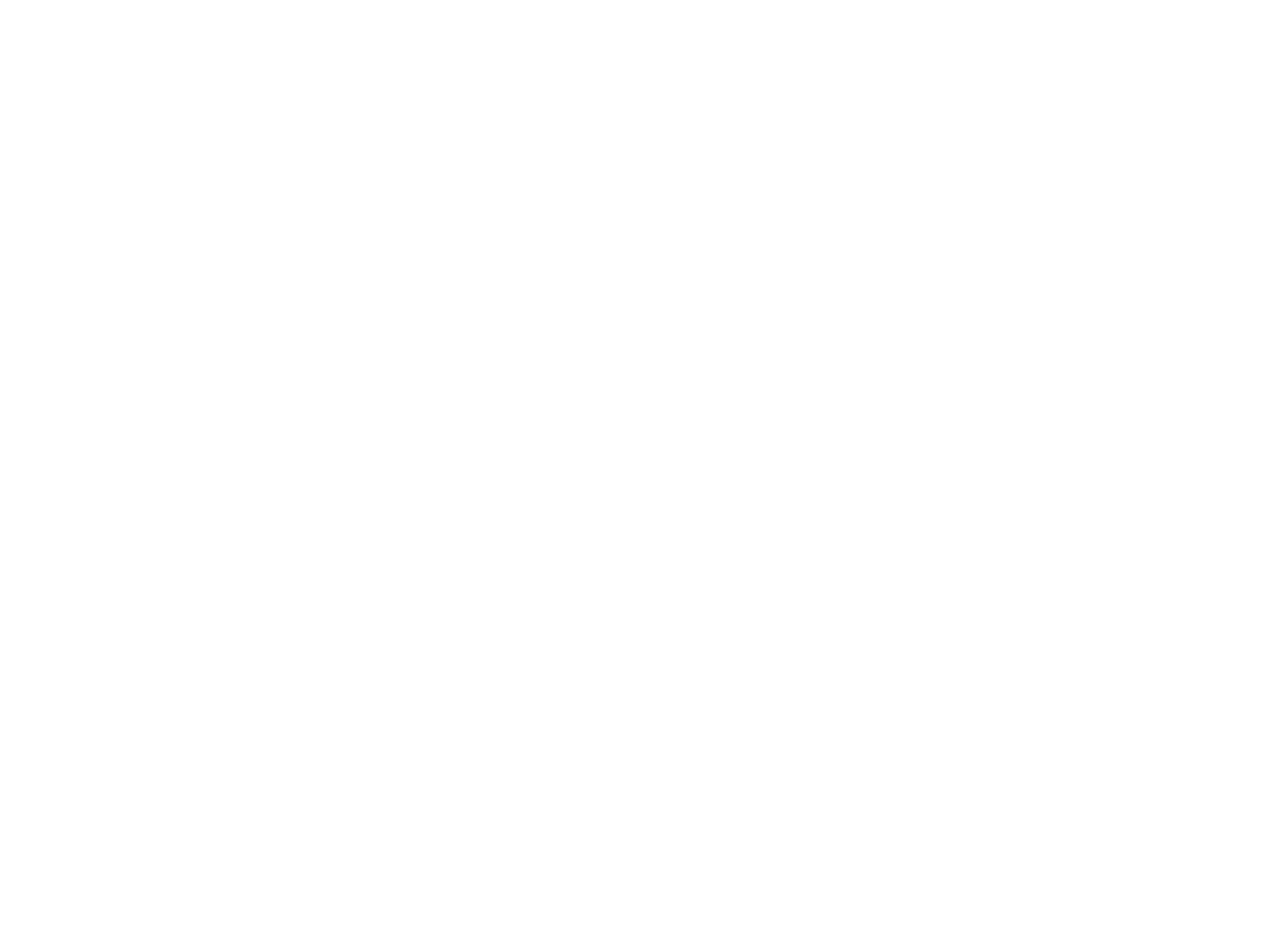
DifferentProgrammingParadigms
• Functional/proceduralprogramming:
– programisalistofinstructionstothecomputer
• Object-orientedprogramming
– programiscomposedofacollectionobjects
that communicate with each other
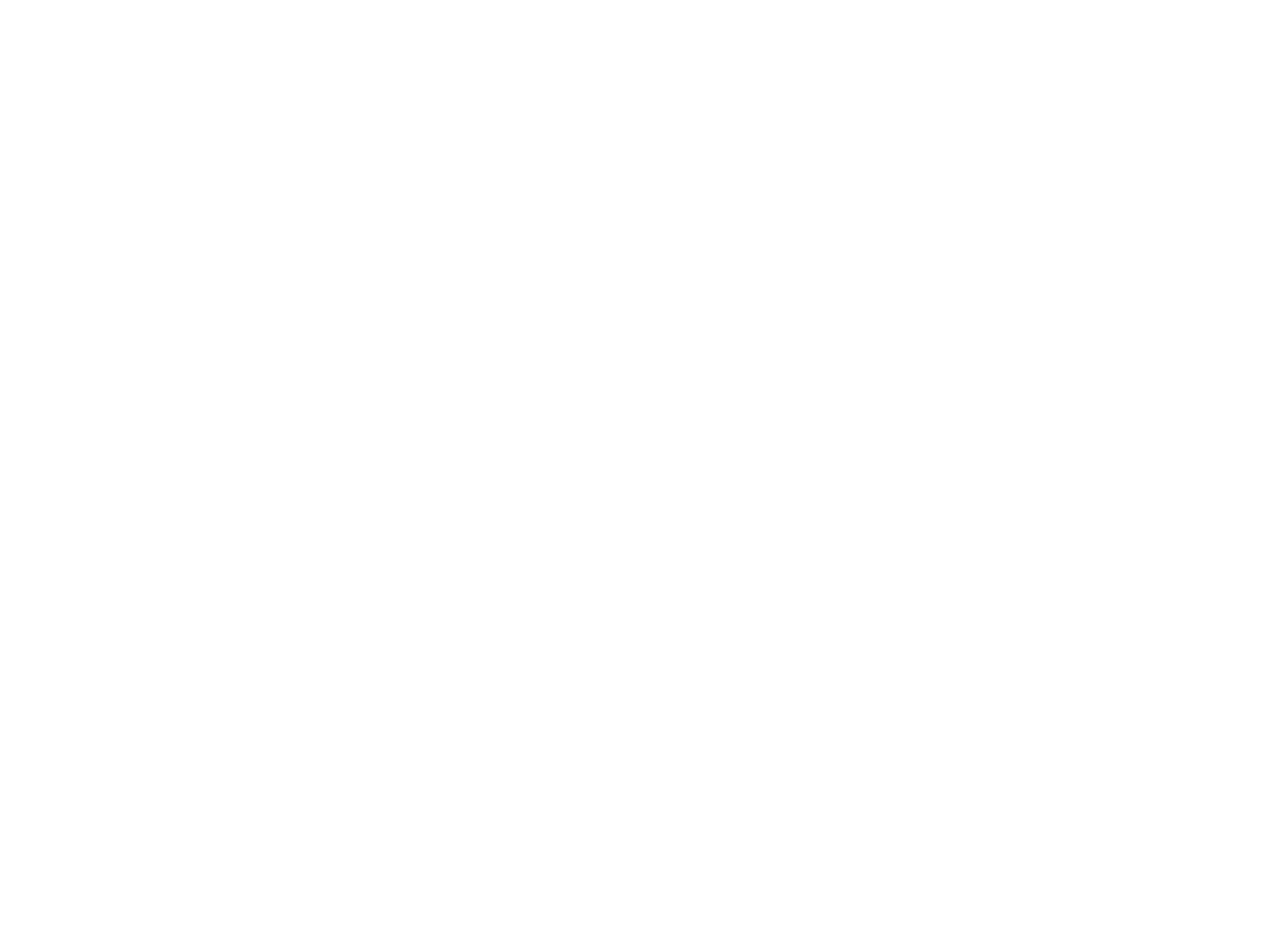
MainConcepts
• Object
• Class
• Inheritance
• Encapsulation
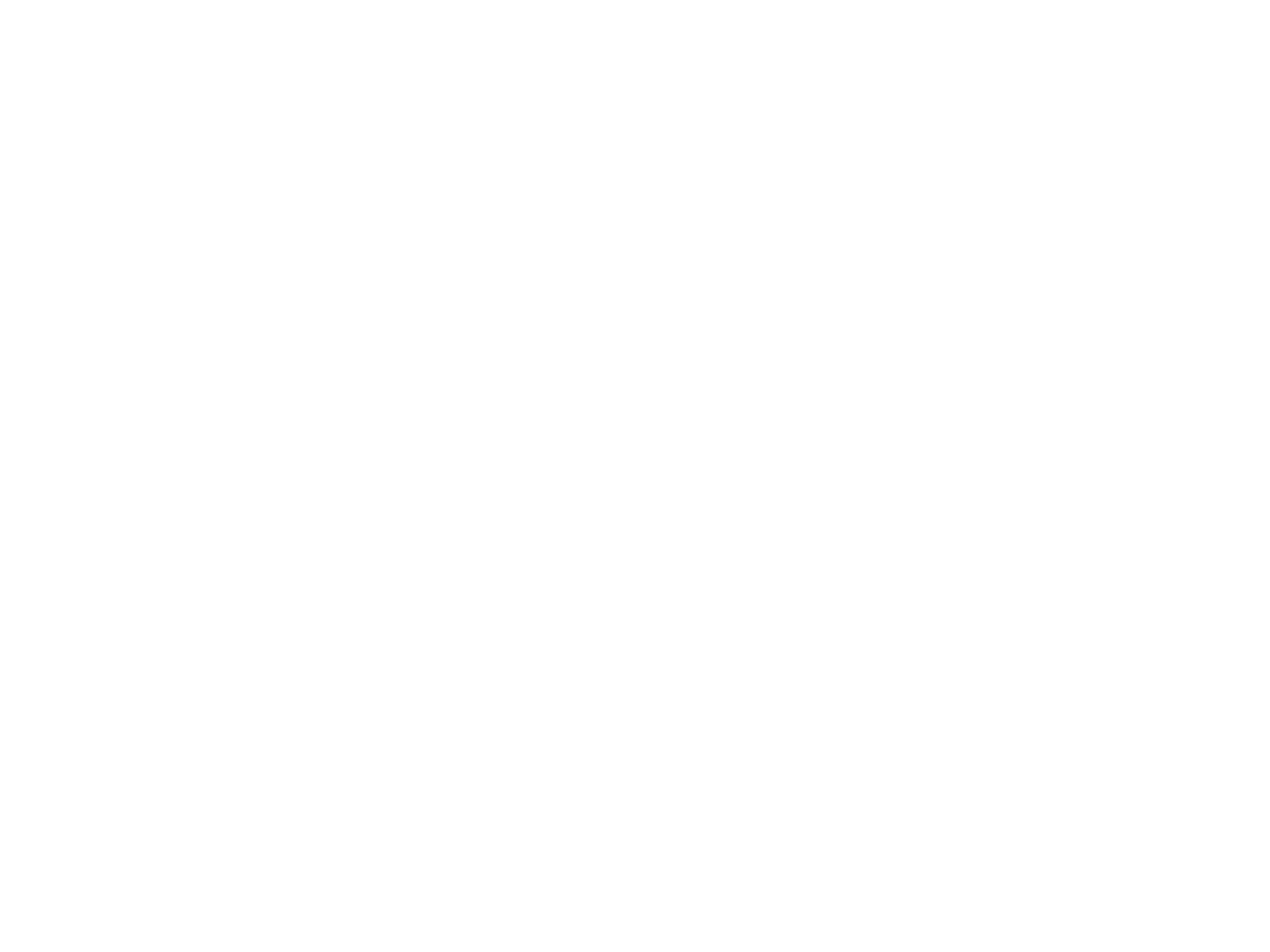
Objects
• identity–uniqueidentificationofanobject
• attributes–data/state
• services–methods/operations
– supportedbytheobject
– withinobjectsresponsibilitytoprovidethese
servicestootherclients
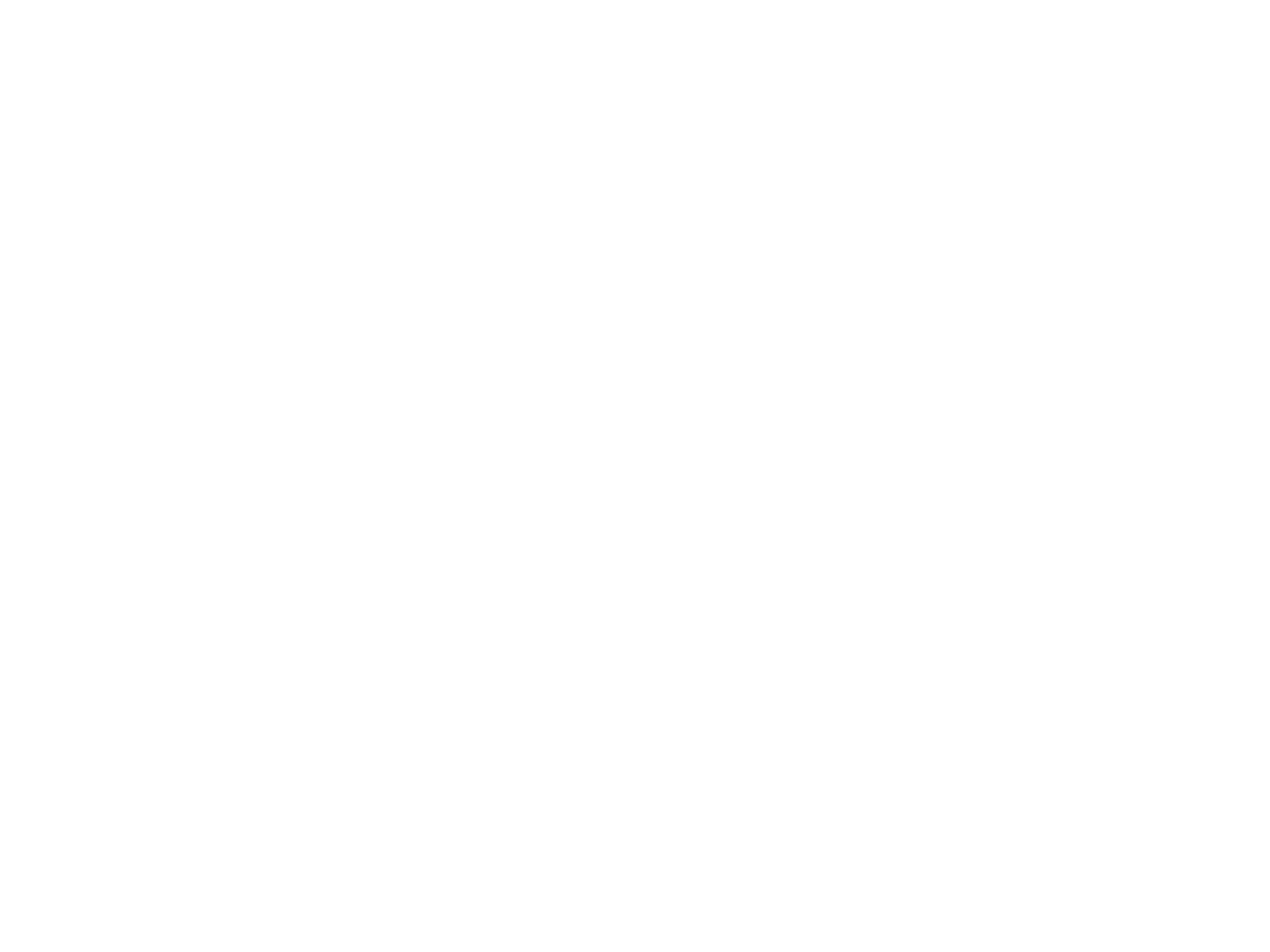
Class
• “type”
• objectisaninstanceofclass
• classgroupssimilarobjects
– same(structureof)attributes
– sameservices
• objectholdsvaluesofitsclass’sattributes
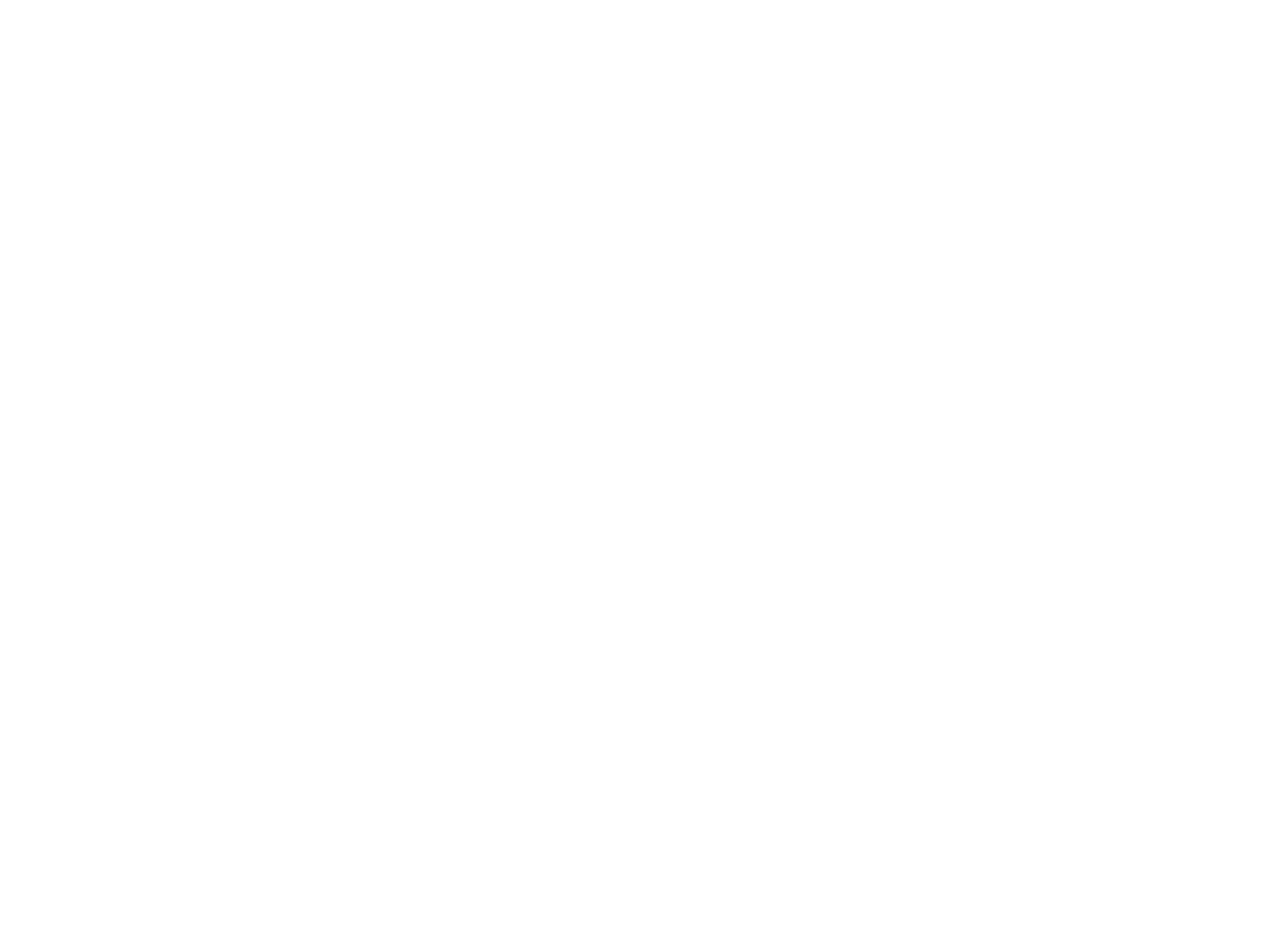
Inheritance
• Classhierarchy
• GeneralizationandSpecialization
– subclassinheritsattributesandservicesfromits
superclass
– subclassmayaddnewattributesandservices
– subclassmayreusethecodeinthesuperclass
– subclassesprovidespecializedbehaviors(overriding
anddynamicbinding)
– partiallydefineandimplementcommonbehaviors
(abstract)
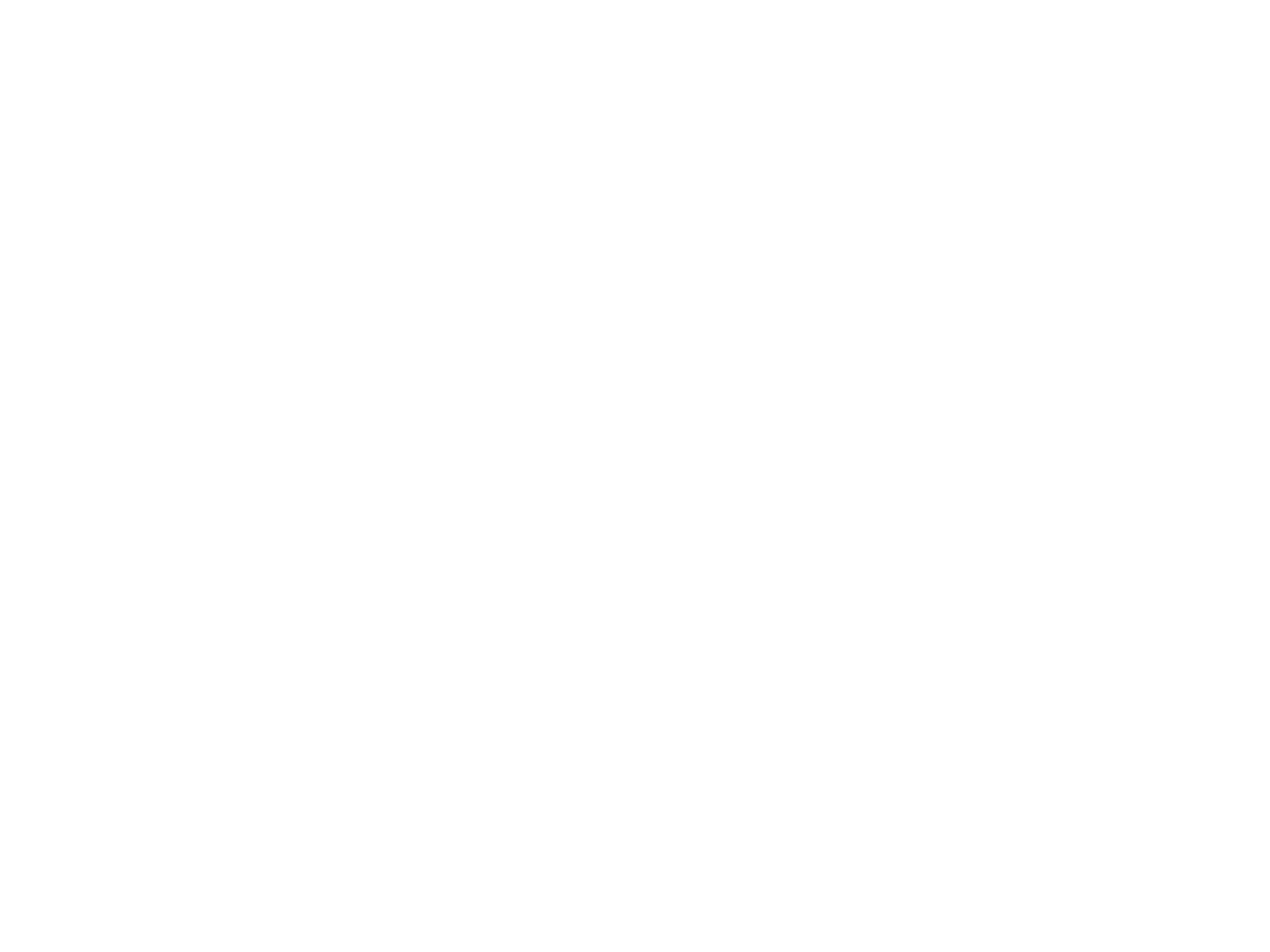
Encapsulation
• Separationbetweeninternalstateoftheobject
anditsexternalaspects
• How?
– controlaccesstomembersoftheclass
– interface“type”
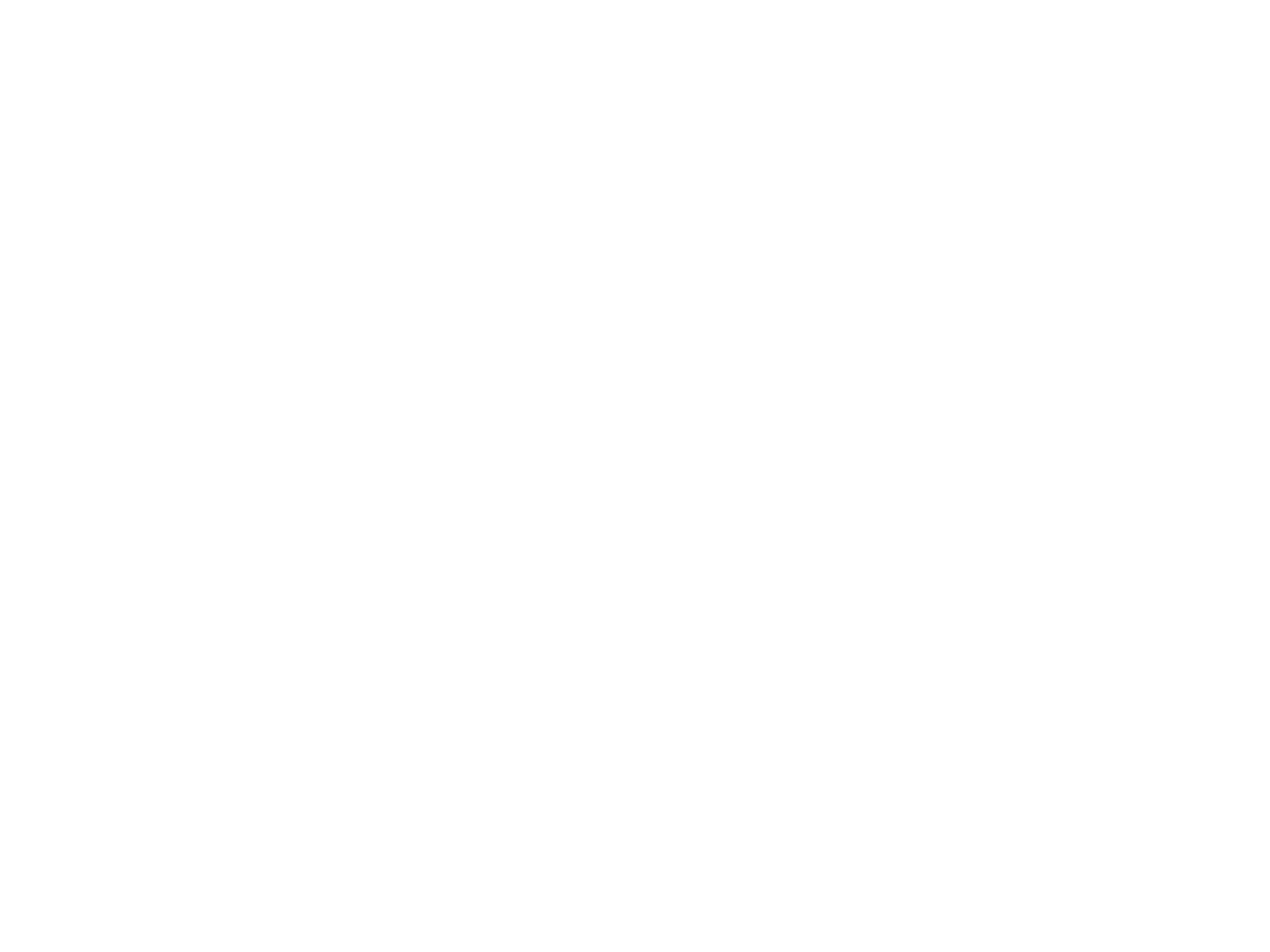
Whatdoesitbuyus?
• Modularity
– sourcecodeforanobjectcanbewrittenandmaintained
independentlyofthesourcecodeforotherobjects
– easiermaintainanceandreuse
• Informationhiding
– otherobjectscanignoreimplementationdetails
– security(objecthascontroloveritsinternalstate)
• but
– shareddataneedspecialdesignpatterns(e.g.,DB)
– performanceoverhead
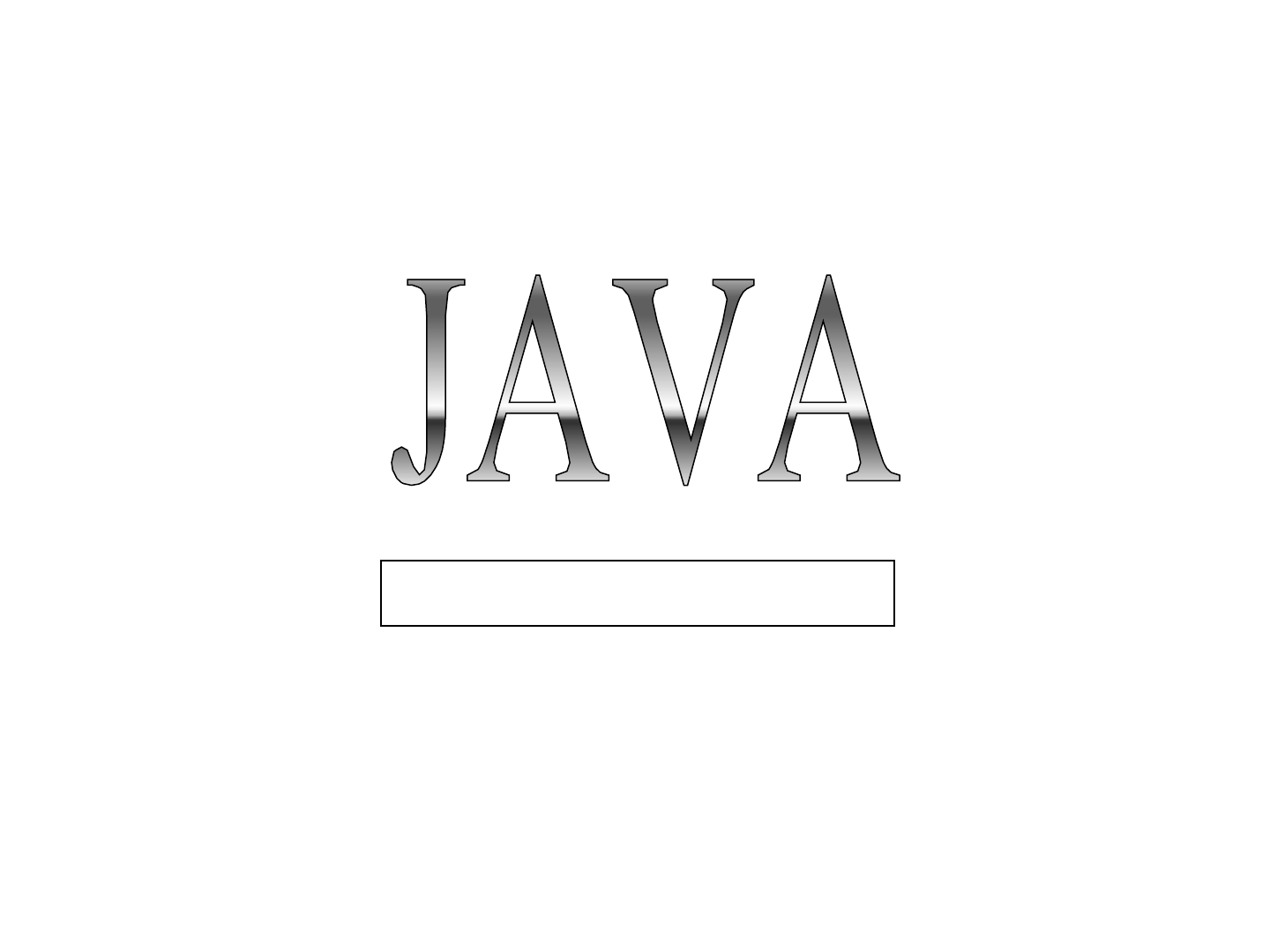
mainly for c++ programmer
AdaptedwithpermissionfromAvivitBercoviciBoden,Technion
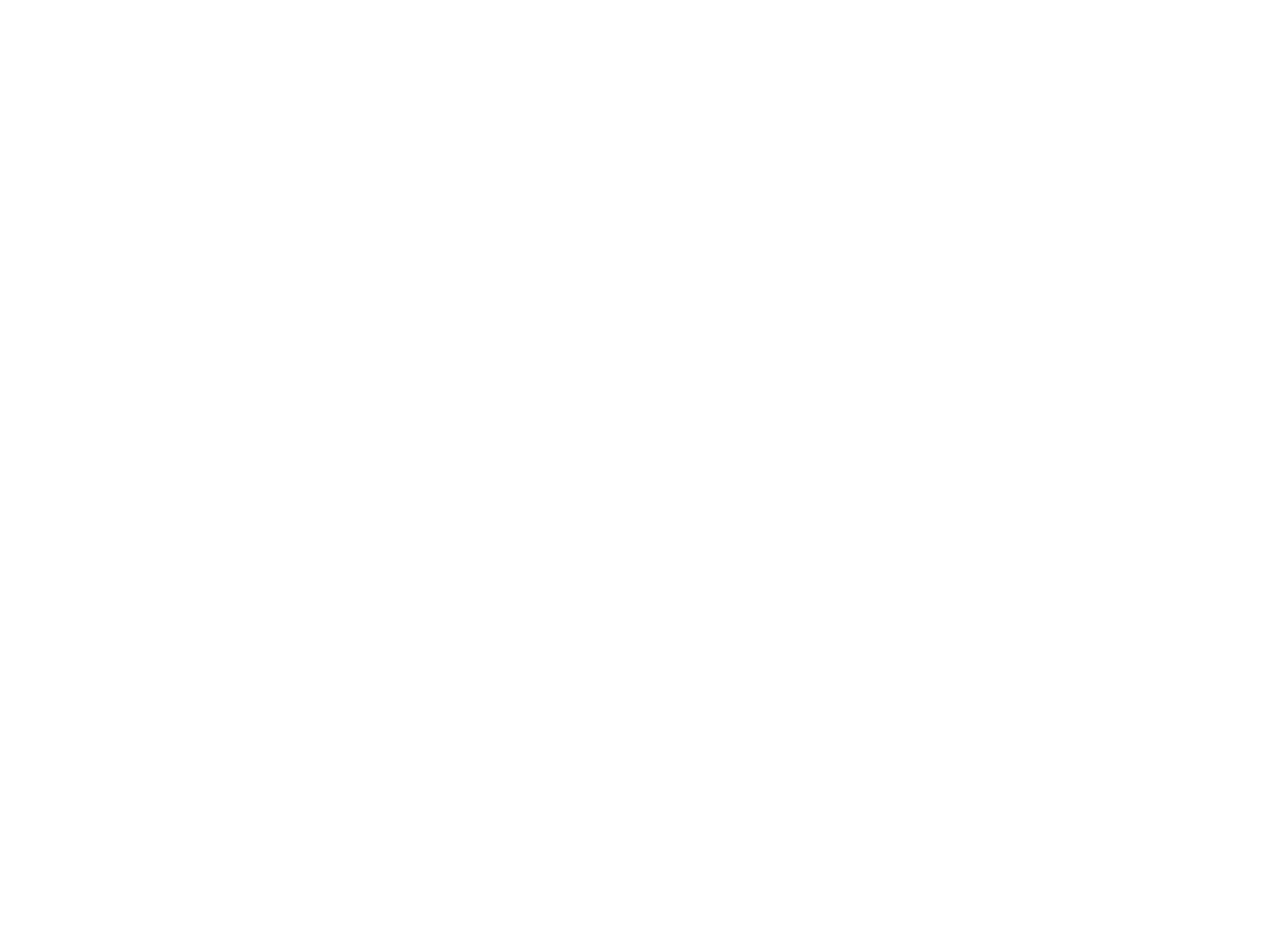
WhyJava?
• Portable
• Easytolearn
• [DesignedtobeusedontheInternet]
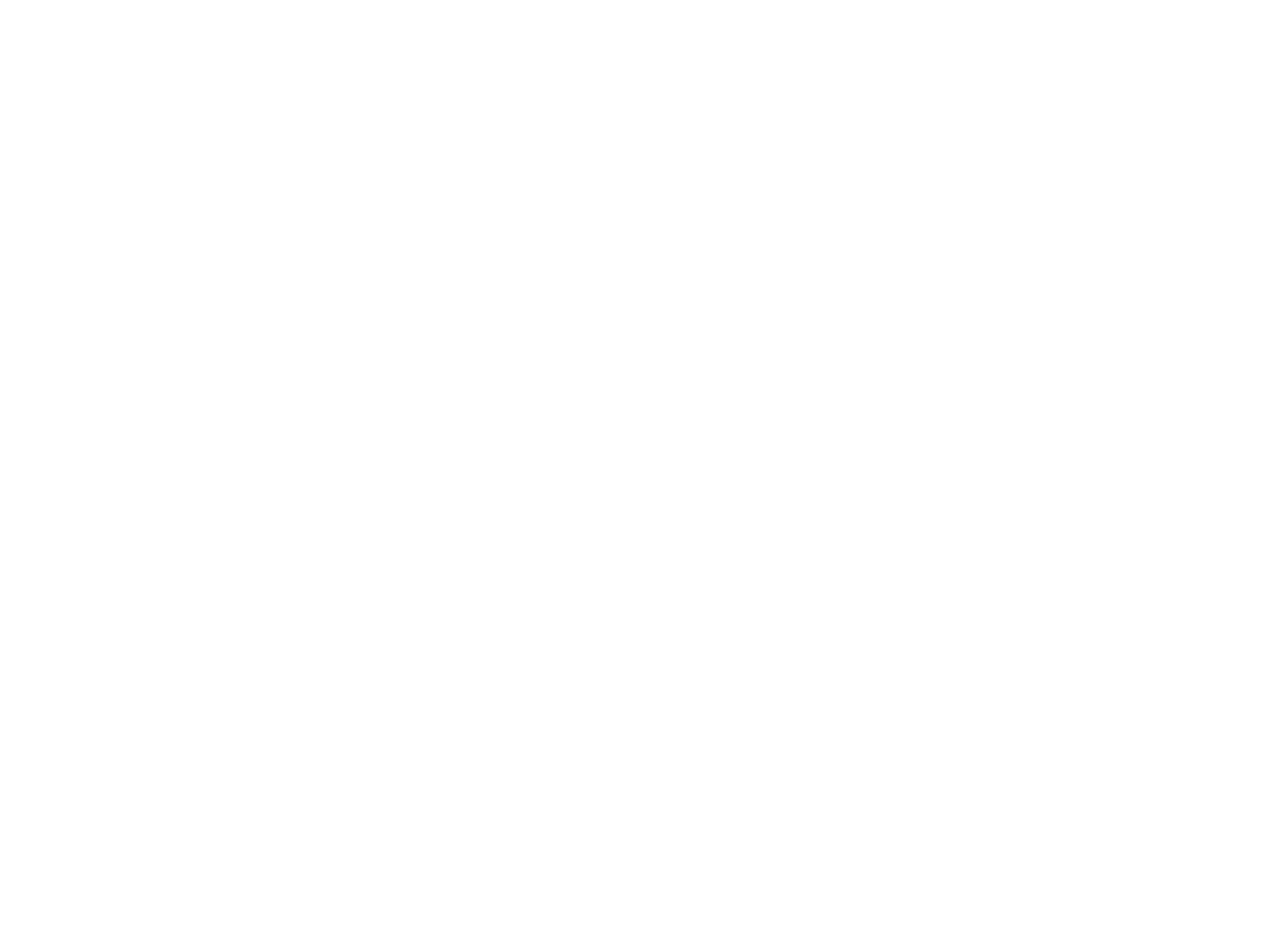
JVM
• JVMstandsfor
Java Virtual Machine
• Unlikeotherlanguages,Java“executables”
areexecutedonaCPUthatdoesnotexist.
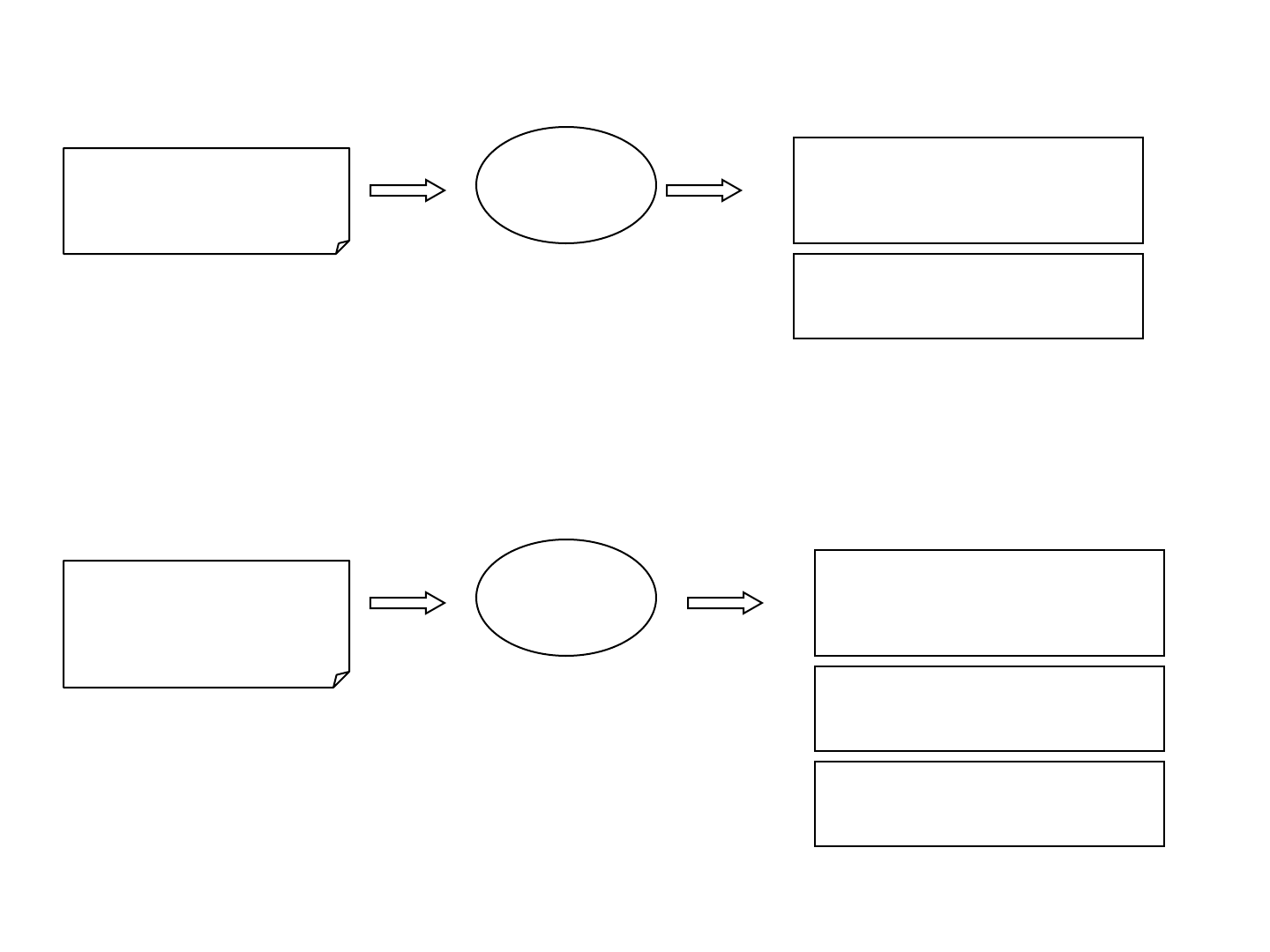
OS/Hardware
machinecode
Csourcecode
myprog.c
gcc
myprog.exe
PlatformDependent
JVM
bytecode
Javasourcecode
myprog.java
javac
myprog.class
OS/Hardware
PlatformIndependent
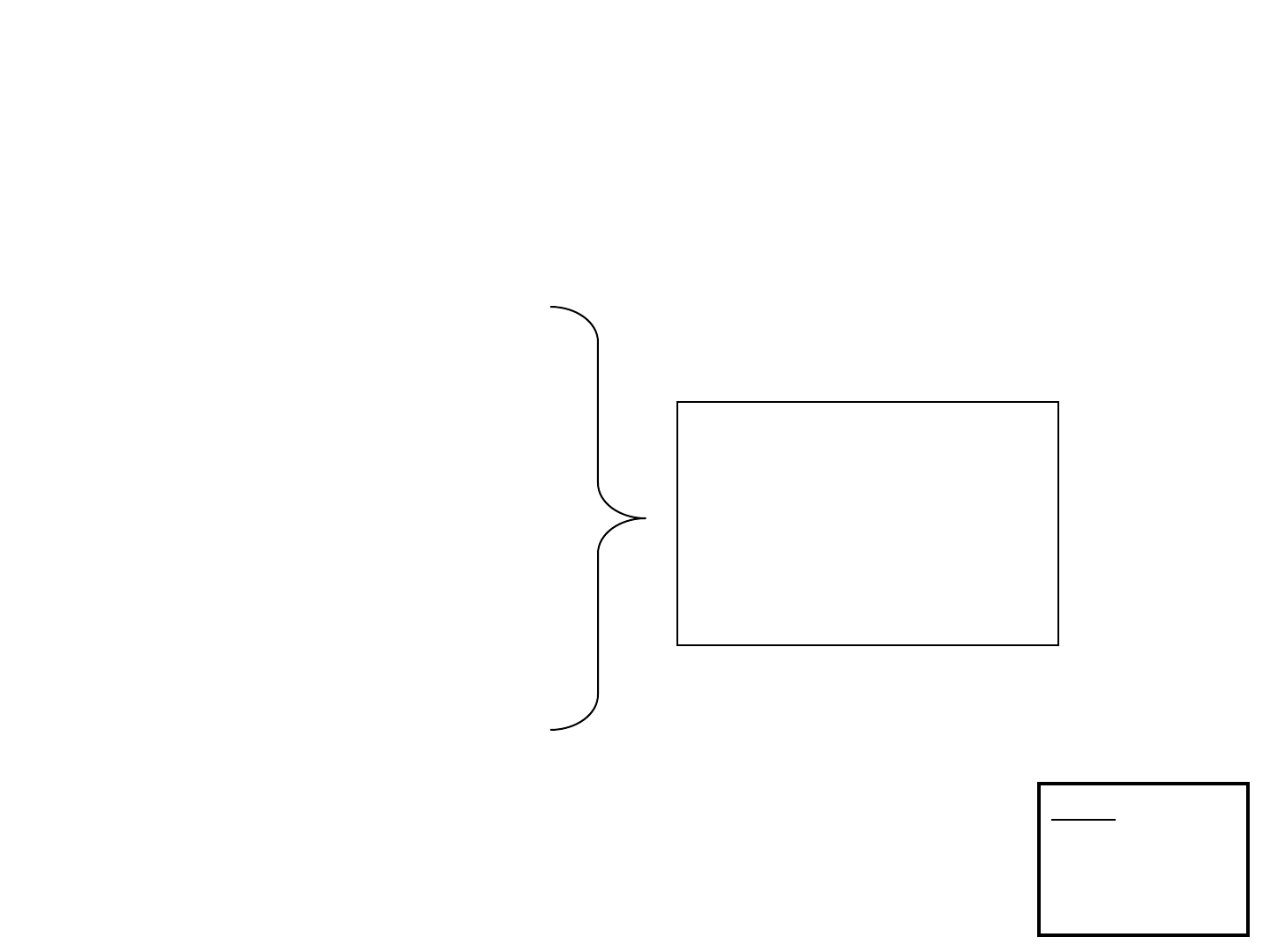
Primitivetypes
• int 4bytes
• short 2bytes
• long 8bytes
• byte 1byte
• float 4bytes
• double 8bytes
• char Unicodeencoding(2bytes)
• boolean{true,false}
Behaviors is
exactly as in
C++
Note:
Primitive type
always begin
with lower-case
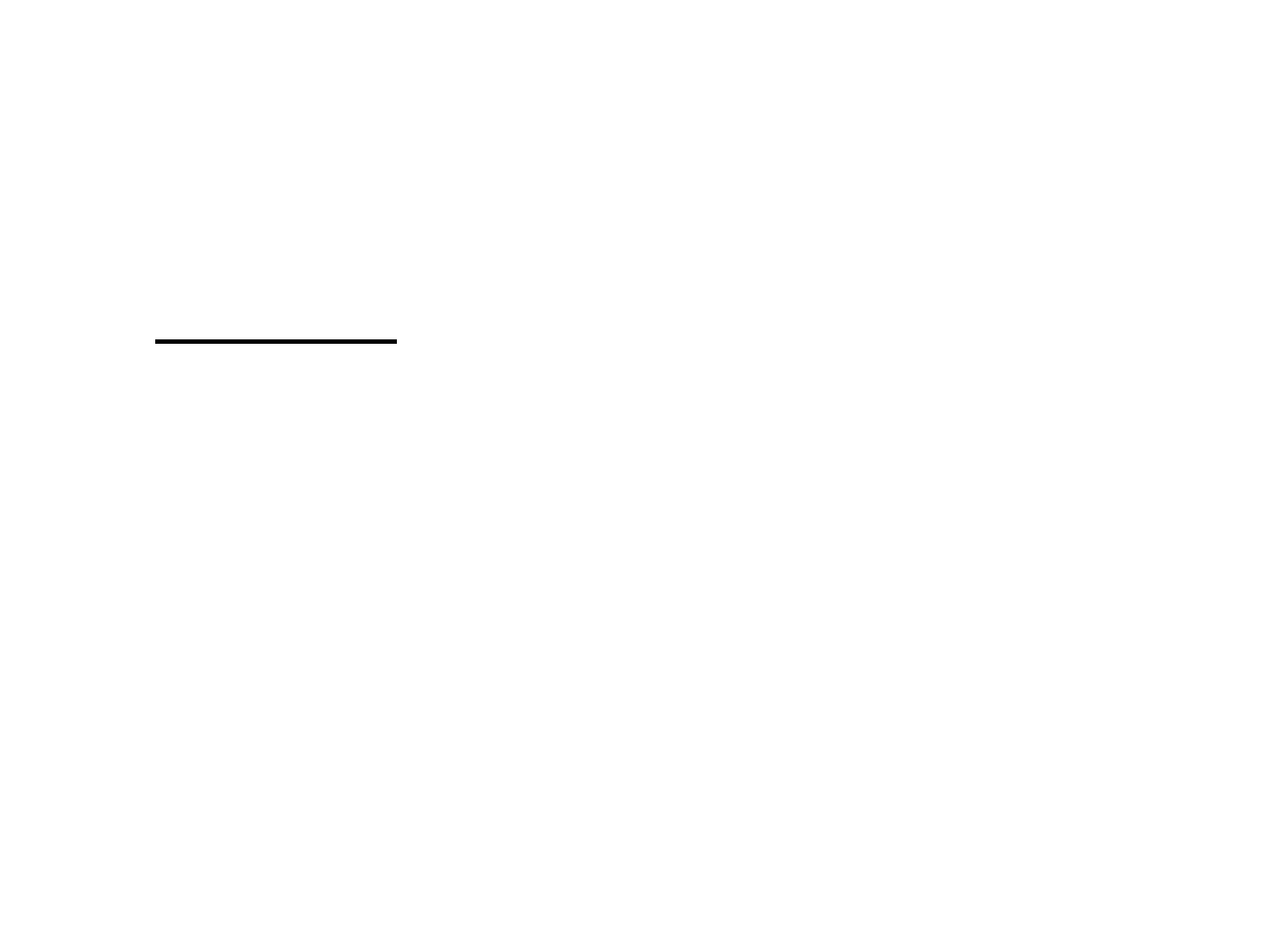
• Constants
37 integer
37.2 float
42F float
0754 integer(octal)
0xfe integer(hexadecimal)
Primitivetypes-cont.
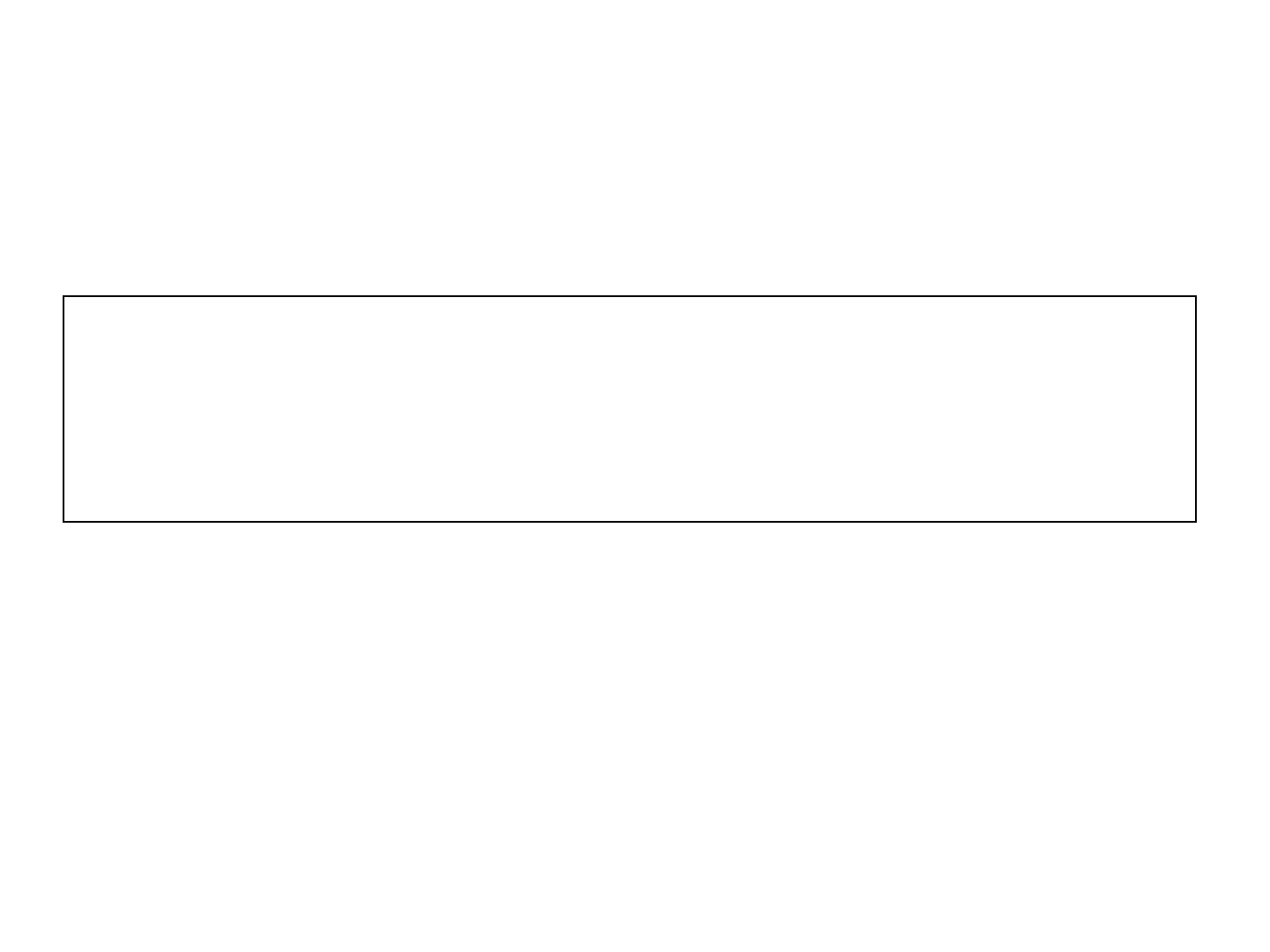
HelloWorld
class Hello {
public static void main(String[] args) {
System.out.println(“Hello World !!!”);
}
}
Hello.java
C:\javacHello.java
C:\javaHello
)compilation creates Hello.class )
(Execution on the local JVM)
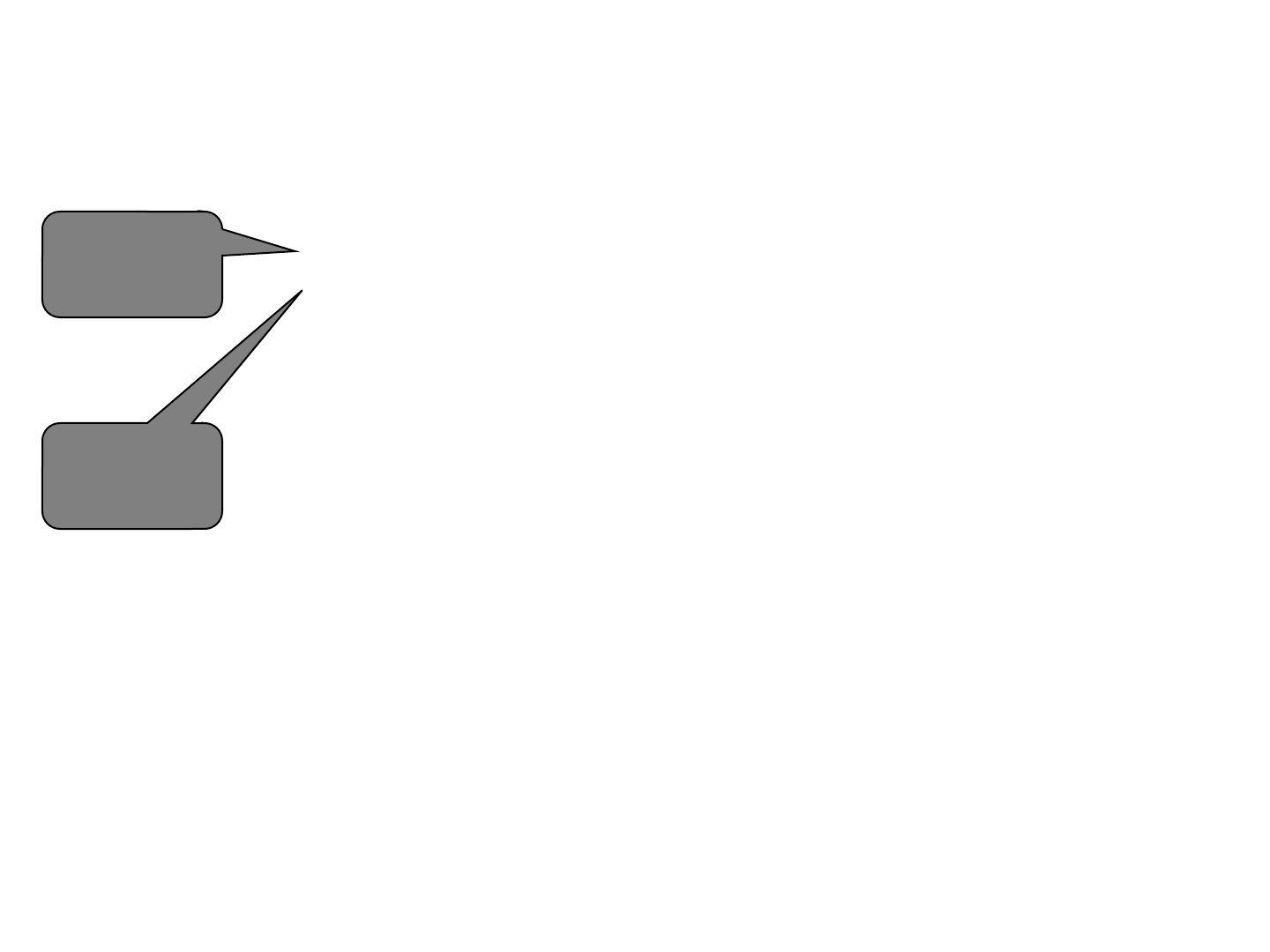
Moresophisticated
class Kyle {
private boolean kennyIsAlive_;
public Kyle() { kennyIsAlive_ = true; }
public Kyle(Kyle aKyle) {
kennyIsAlive_ = aKyle.kennyIsAlive_;
}
public String theyKilledKenny() {
if (kennyIsAlive_) {
kennyIsAlive_ = false;
return “You bastards !!!”;
} else {
return “?”;
}
}
public static void main(String[] args) {
Kyle k = new Kyle();
String s = k.theyKilledKenny();
System.out.println(“Kyle: “ + s);
}
}
Default
C’tor
Copy
C’tor
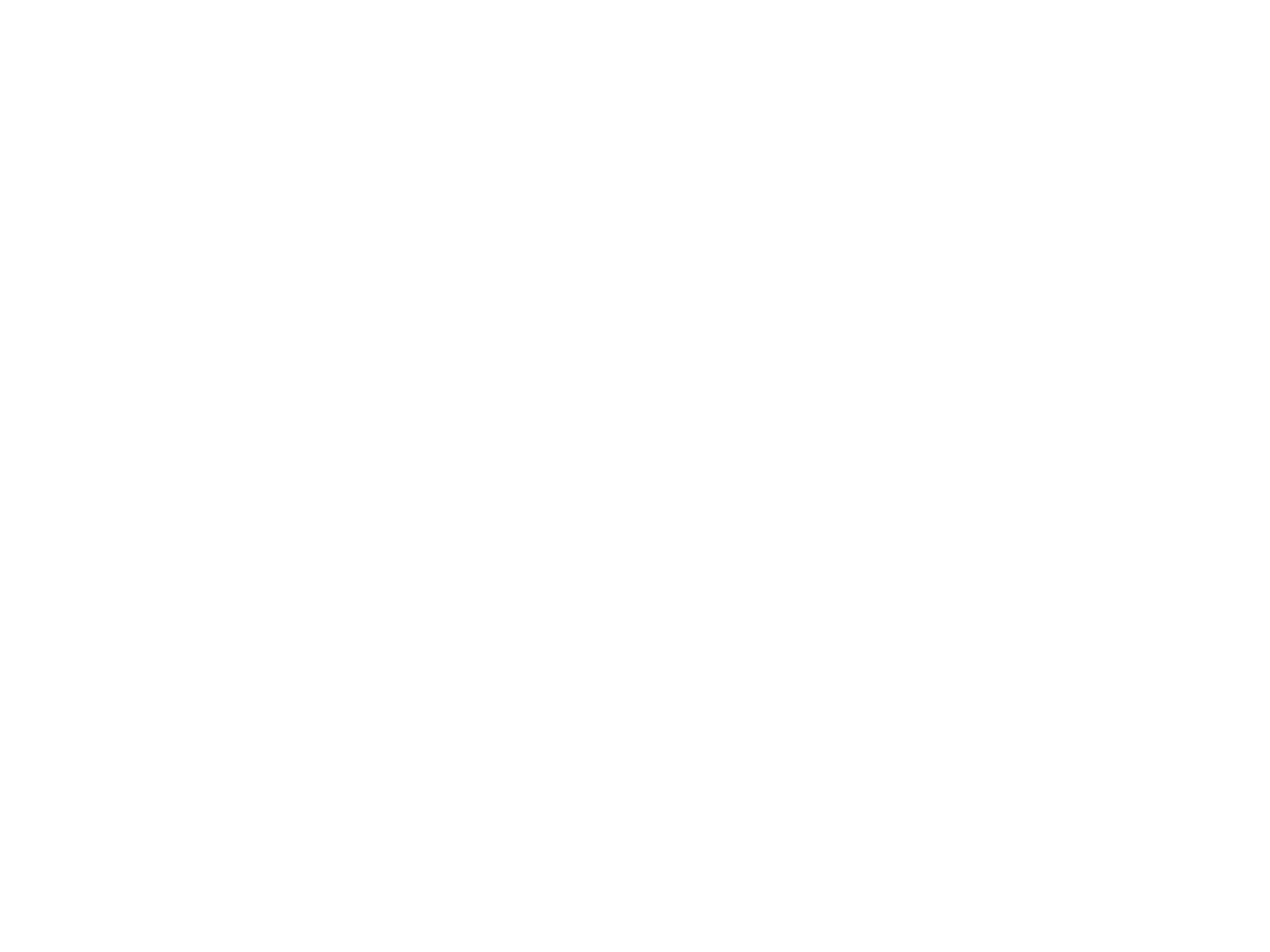
Results
javac Kyle.java ( to compile )
java Kyle ( to execute )
Kyle: You bastards !!!
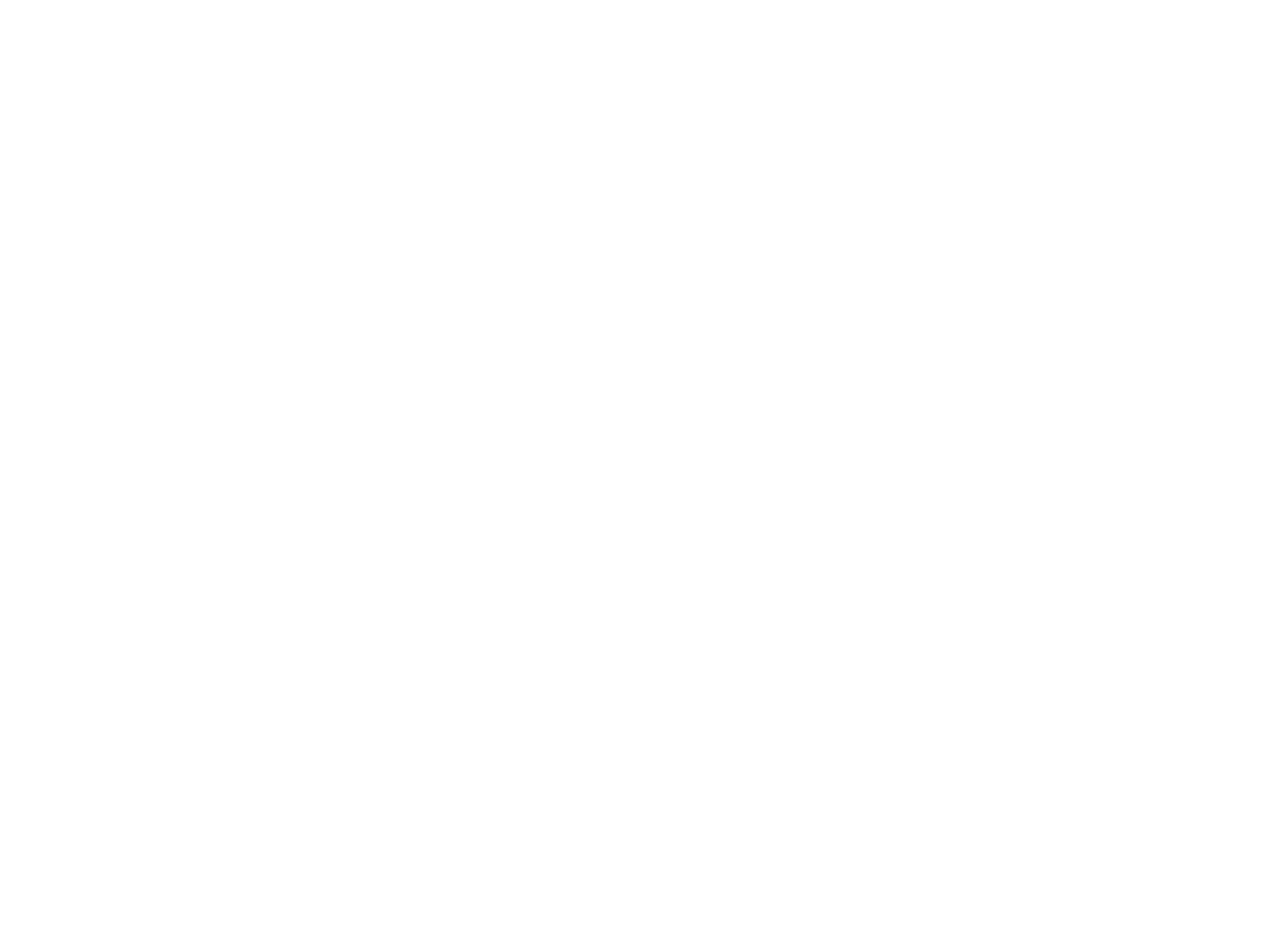
Arrays
•Arrayisanobject
•Arraysizeisfixed
Animal[] arr; // nothing yet …
arr = new Animal[4]; // only array of pointers
for(int i=0 ; i < arr.length ; i++) {
arr[i] = new Animal();
// now we have a complete array
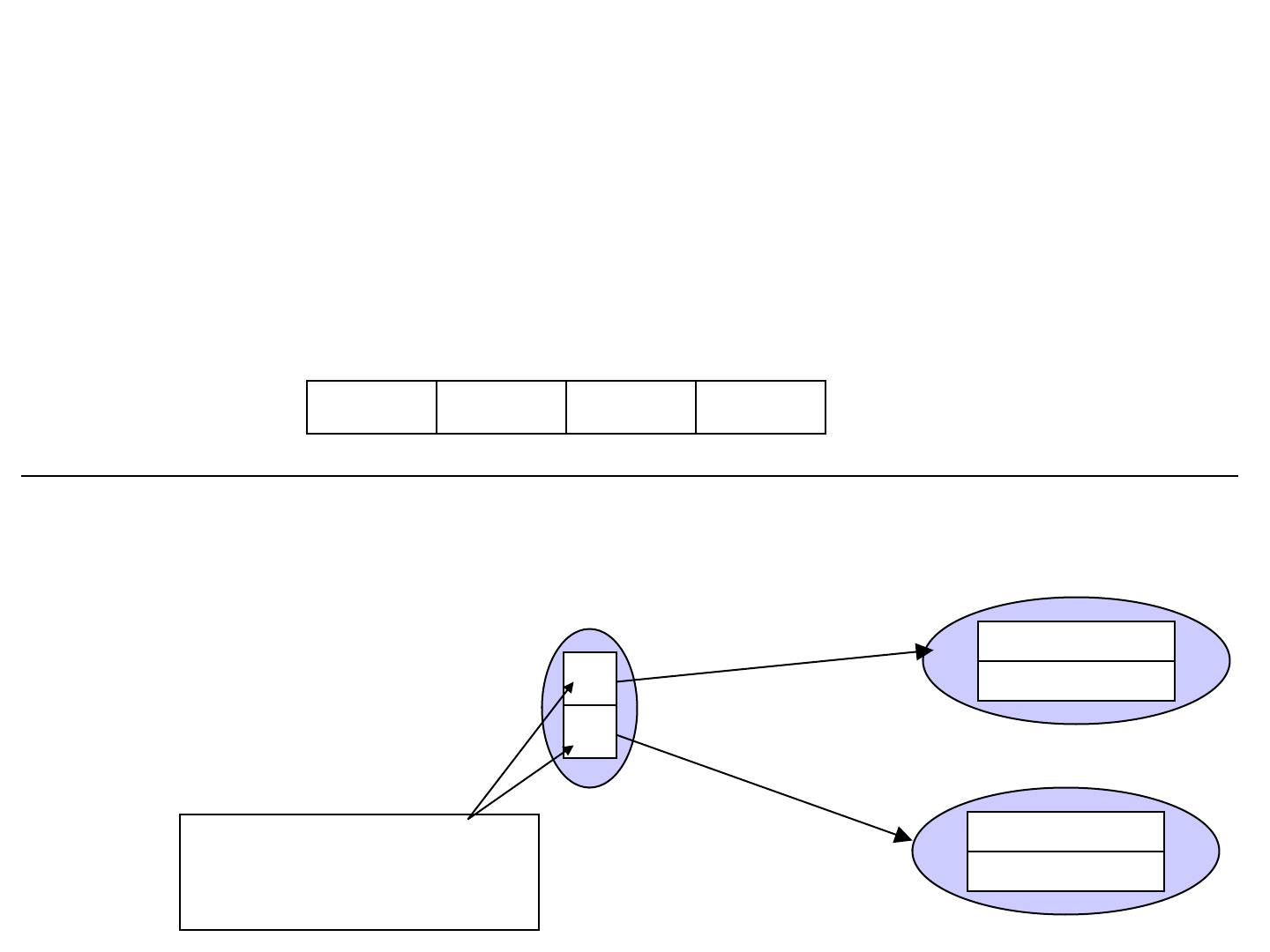
Arrays-Multidimensional
• InC++
Animal arr[2][2]
Is:
• InJava
Whatisthetypeof
theobjecthere?
Animal[][] arr=
new Animal[2][2]
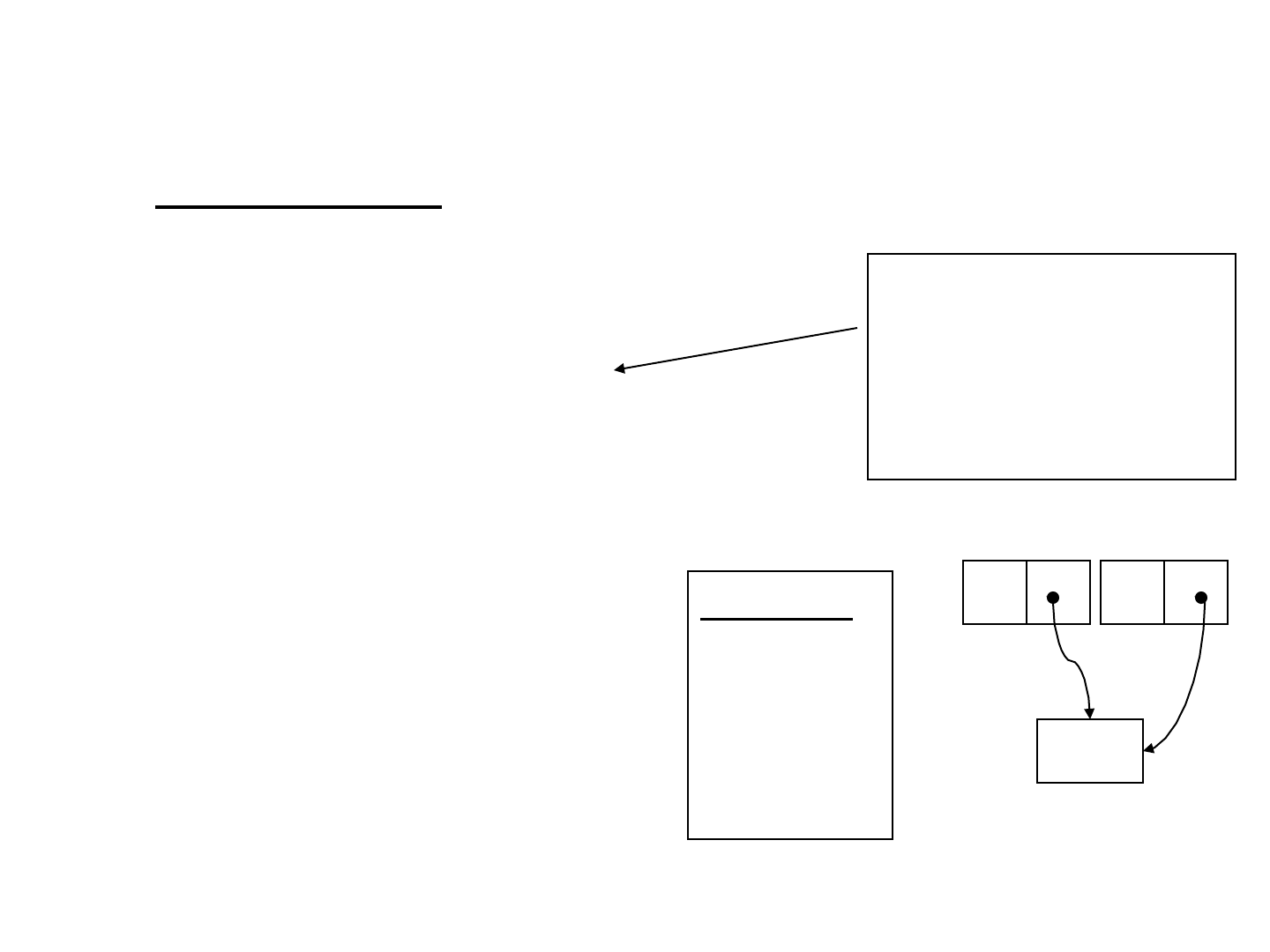
Static-[1/4]
• Member data-Samedataisusedforallthe
instances(objects)ofsomeClass.
Class A {
public int y = 0;
public static int x_ = 1;
};
A a = new A();
A b = new A();
System.out.println(b.x_);
a.x_ = 5;
System.out.println(b.x_);
A.x_ = 10;
System.out.println(b.x_);
Assignment performed
on the first access to the
Class.
Only one instance of ‘x’
exists in memory
Output:
1
5
10
a b
y y
A.x_
0 0
1
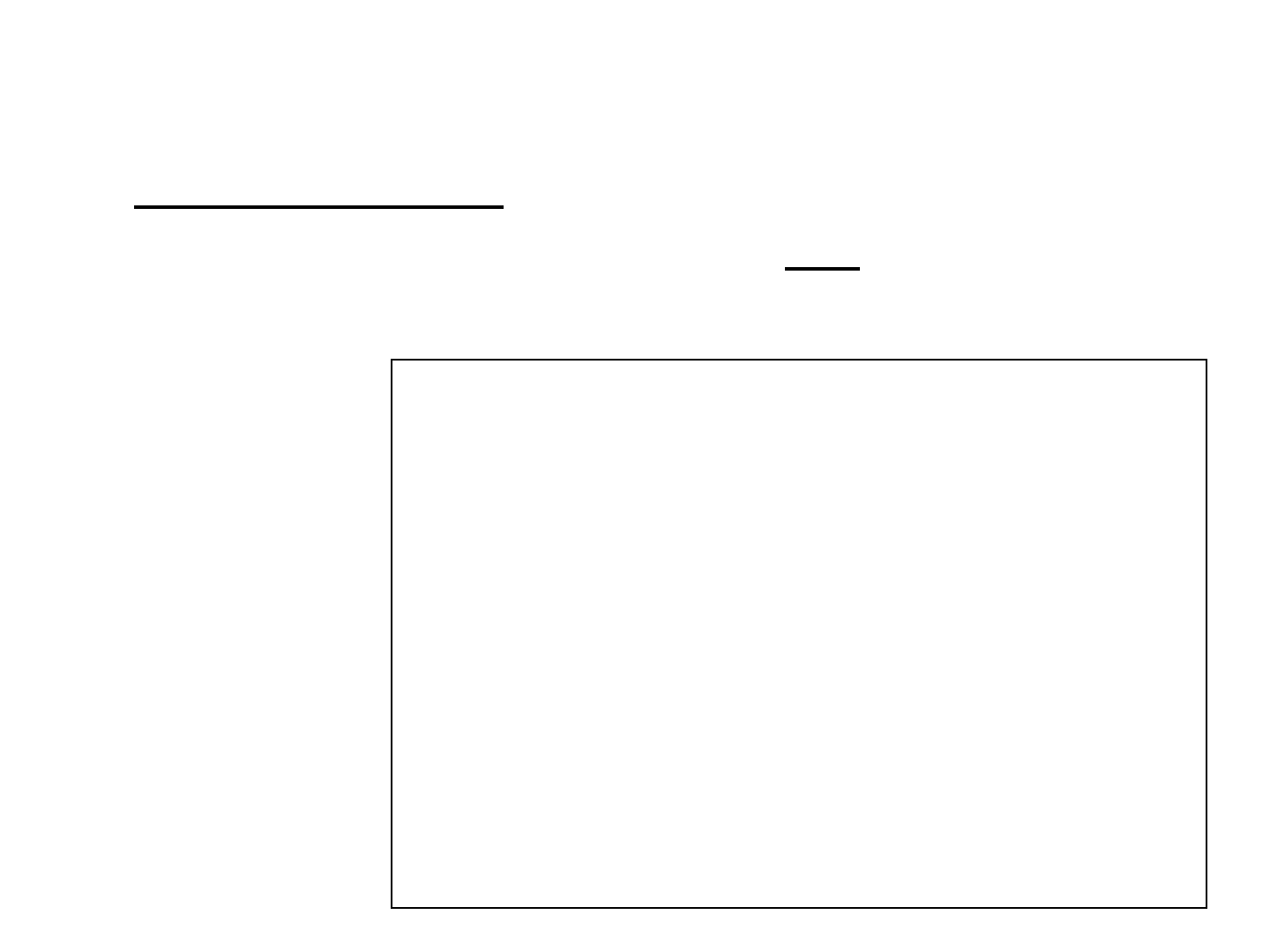
Static-[2/4]
• Member function
– Staticmemberfunctioncanaccessonlystaticmembers
– Staticmemberfunctioncanbecalledwithoutan
instance.
Class TeaPot {
private static int numOfTP = 0;
private Color myColor_;
public TeaPot(Color c) {
myColor_ = c;
numOfTP++;
}
public static int howManyTeaPots()
{ return numOfTP; }
// error :
public static Color getColor()
{ return myColor_; }
}
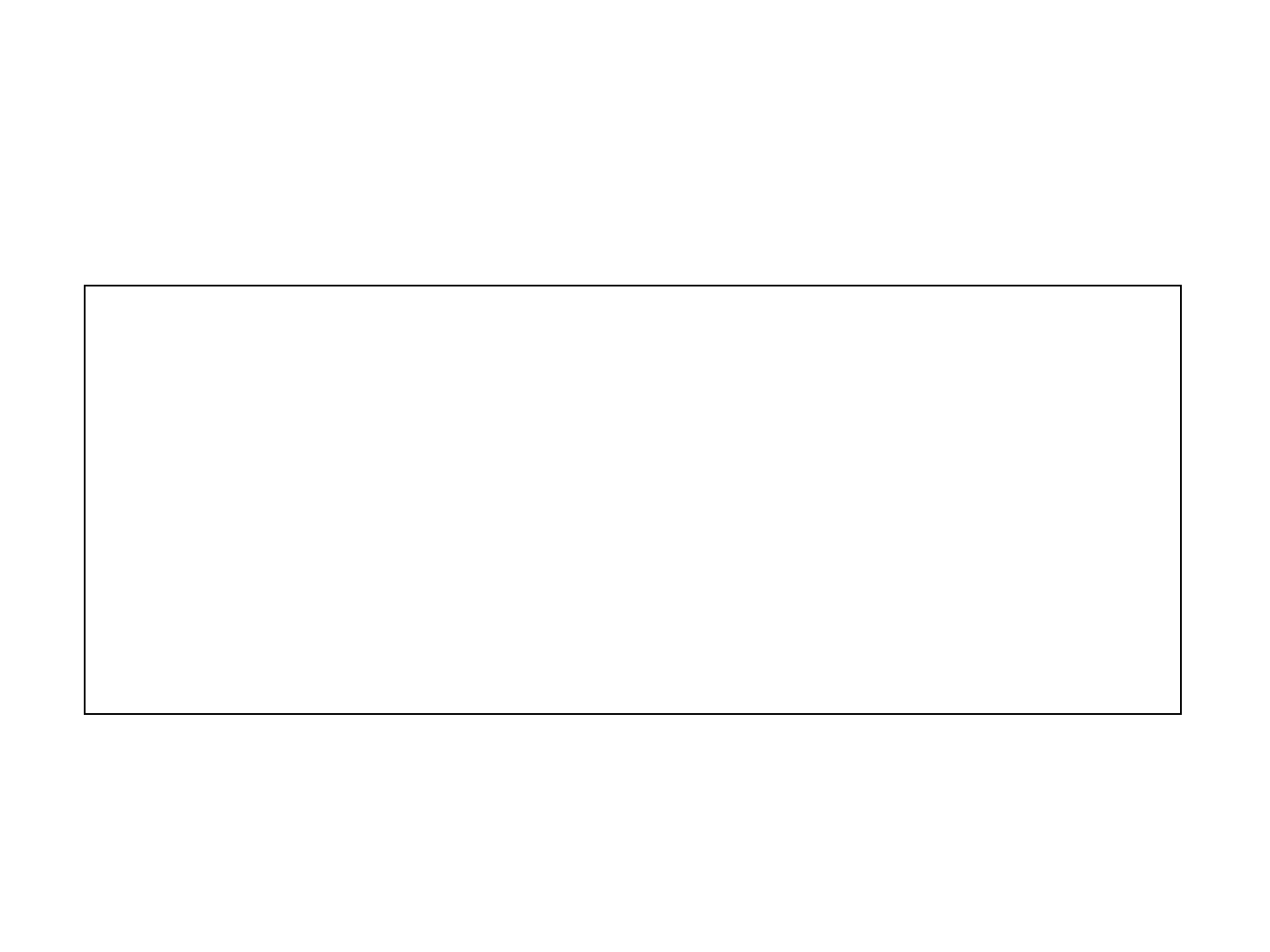
Static-[2/4]cont.
Usage:
TeaPot tp1 = new TeaPot(Color.RED);
TeaPot tp2 = new TeaPot(Color.GREEN);
System.out.println(“We have “ +
TeaPot.howManyTeaPots()+ “Tea Pots”);
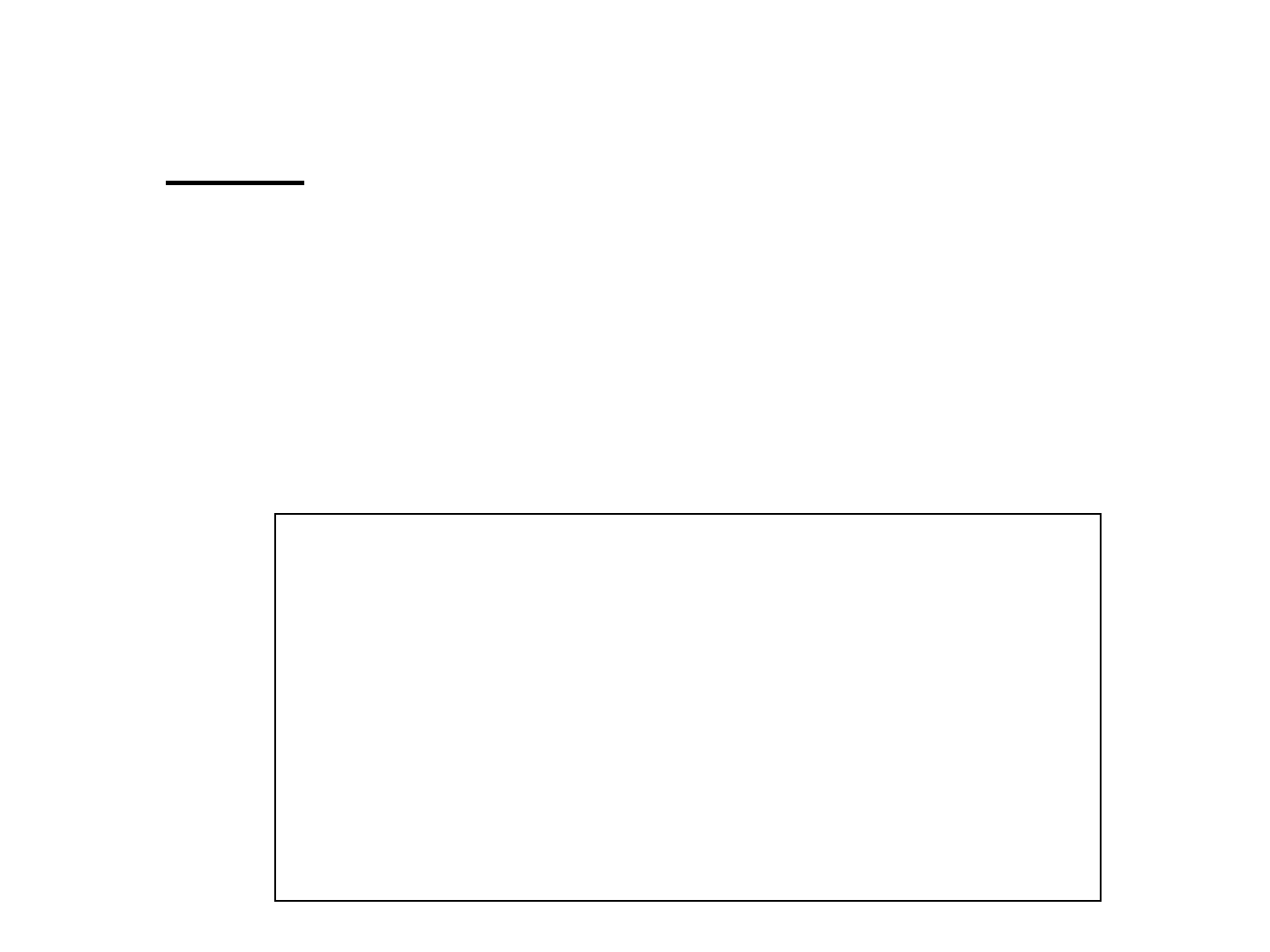
Static-[3/4]
• Block
– Codethatisexecutedinthefirstreferencetotheclass.
– Severalstaticblockscanexistinthesameclass
(Executionorderisbytheappearanceorderintheclass
definition).
– Onlystaticmemberscanbeaccessed.
class RandomGenerator {
private static int seed_;
static {
int t = System.getTime() % 100;
seed_ = System.getTime();
while(t-- > 0)
seed_ = getNextNumber(seed_);
}
}
}
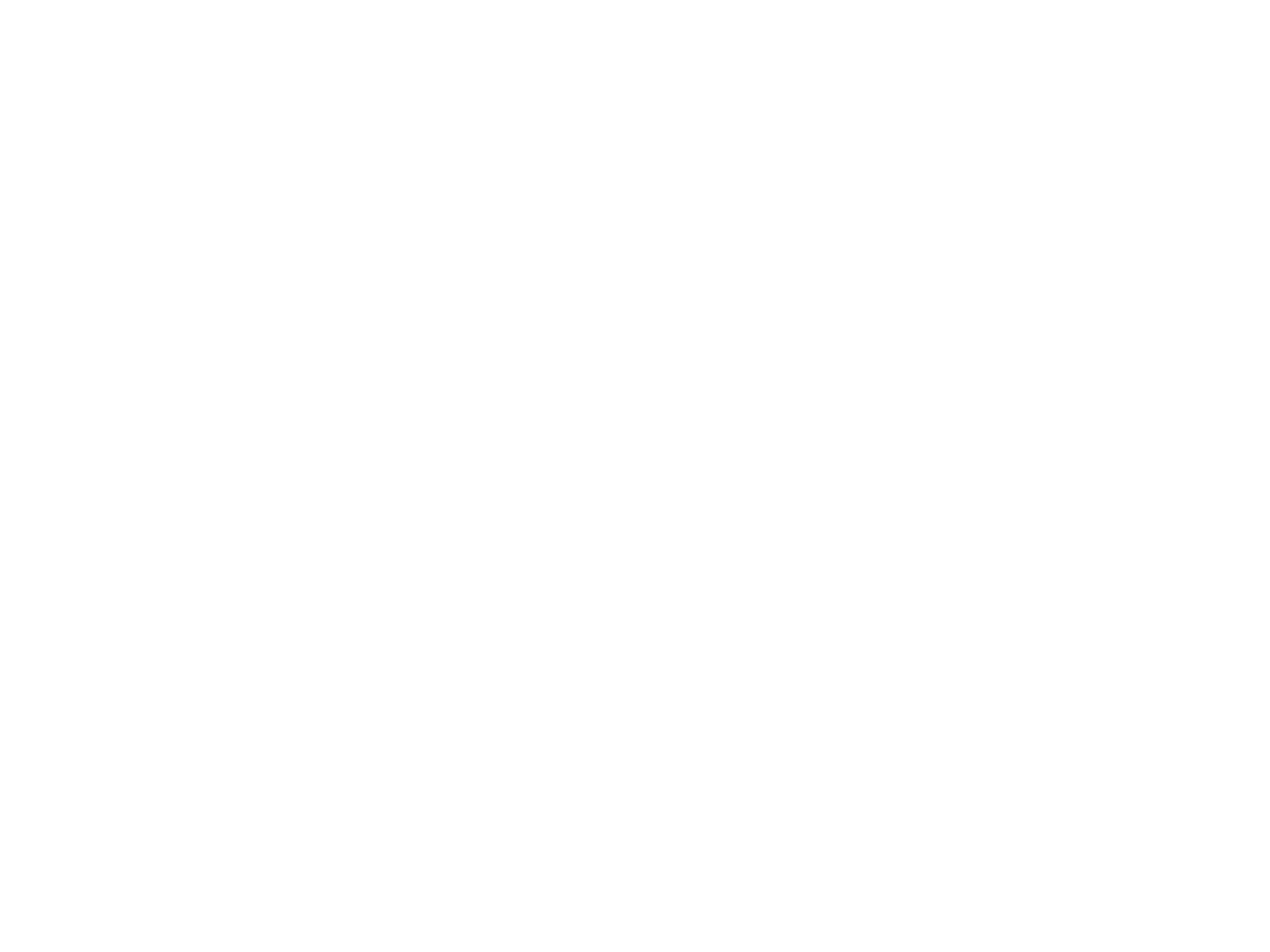
StringisanObject
•ConstantstringsasinC,doesnotexist
•Thefunctioncallfoo(“Hello”)createsaStringobject,
containing“Hello”,andpassesreferencetoittofoo.
•Thereisnopointinwriting:
•TheStringobjectisaconstant.Itcan’tbechangedusing
areferencetoit.
String s = new String(“Hello”);
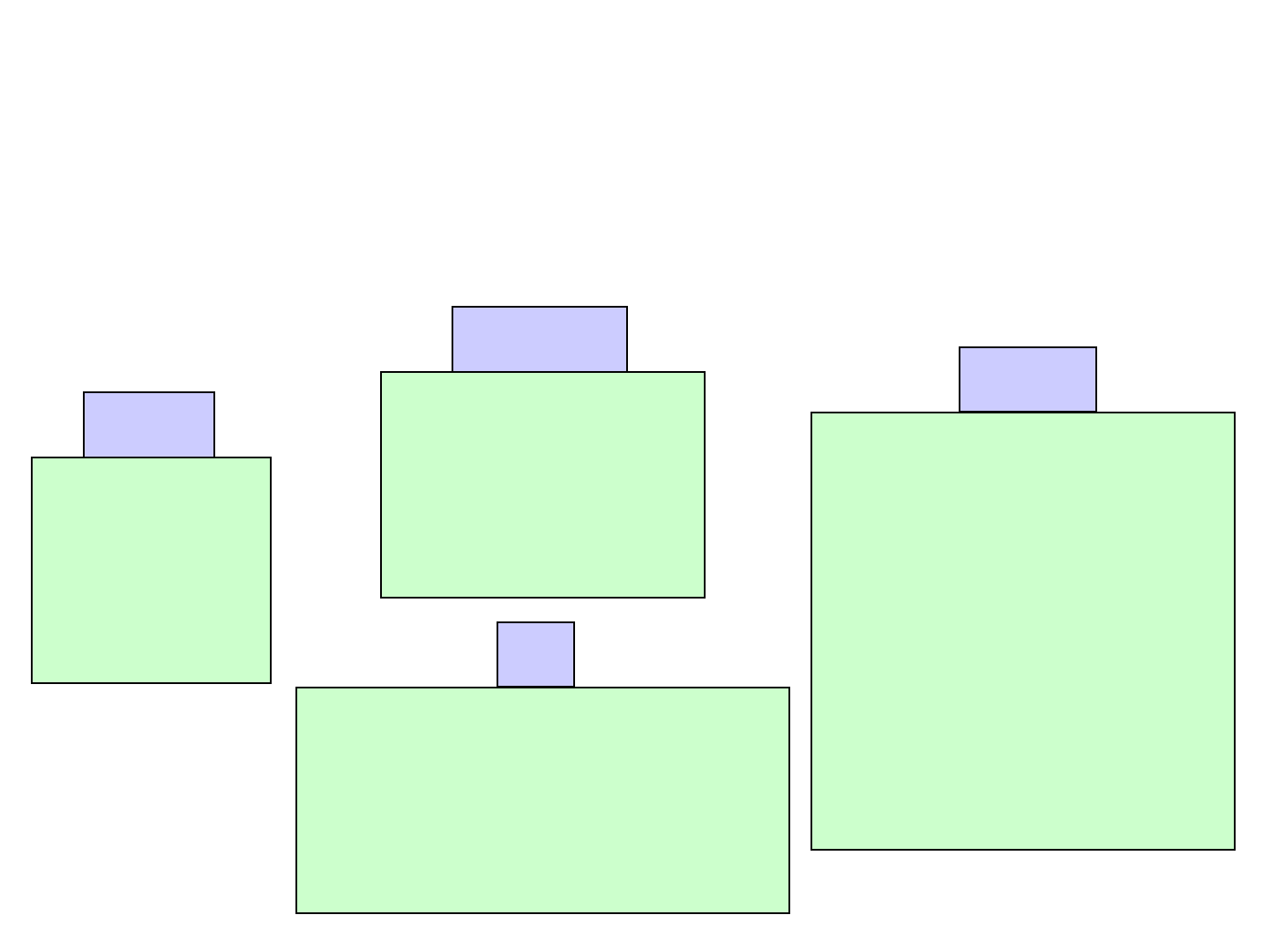
Flowcontrol
Basically,itisexactlylikec/c++.
if/else
do/while
for
switch
If(x==4) {
// act1
} else {
// act2
}
int i=5;
do {
// act1
i--;
} while(i!=0);
int j;
for(int i=0;i<=9;i++)
{
j+=i;
}
char
c=IN.getChar();
switch(c) {
case ‘a’:
case ‘b’:
// act1
break;
default:
// act2
}
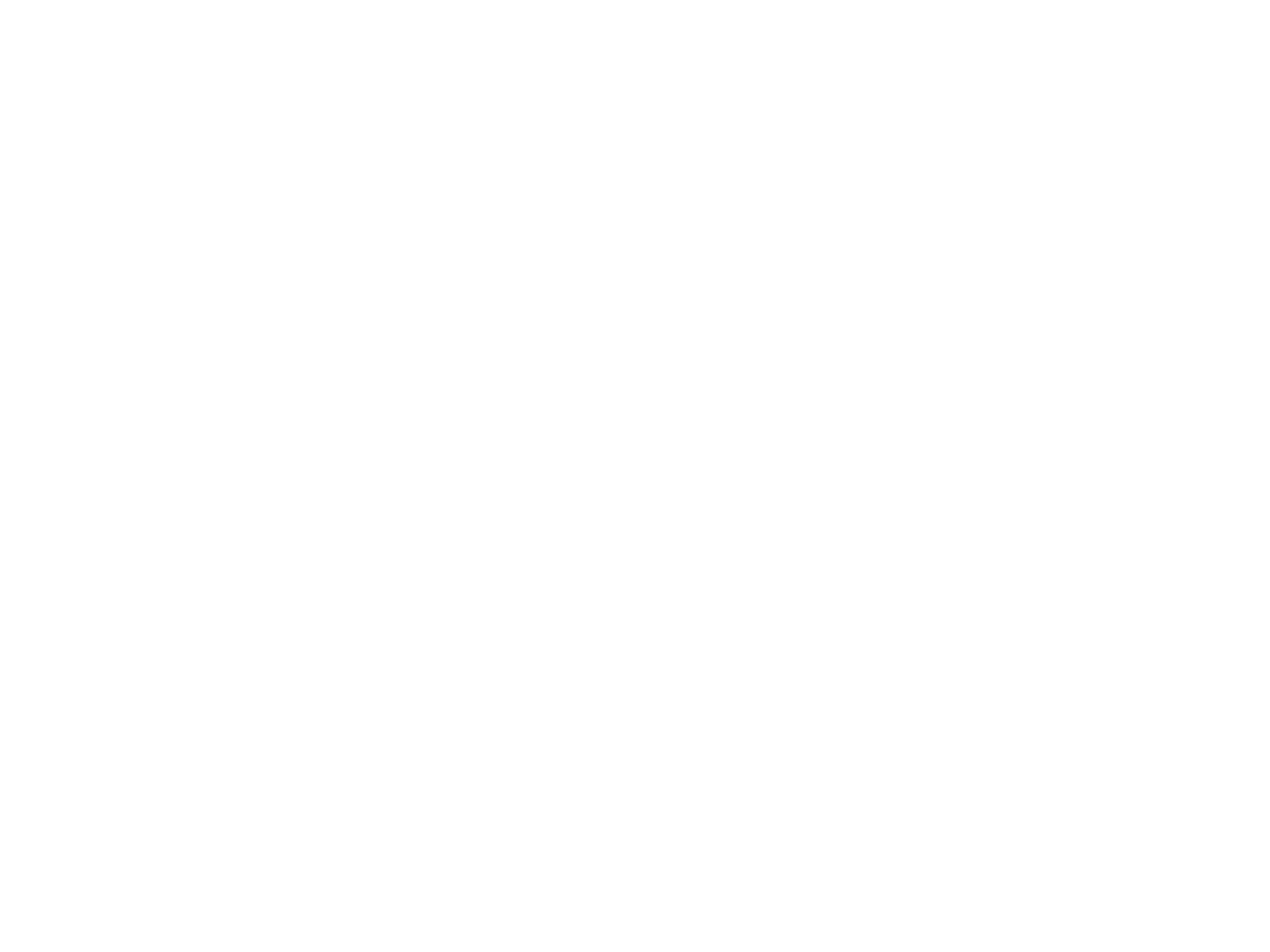
Packages
• Javacodehashierarchicalstructure.
• TheenvironmentvariableCLASSPATHcontains
thedirectorynamesoftheroots.
• EveryObjectbelongstoapackage(‘package’
keyword)
• Objectfullnamecontainsthenamefullnameofthe
packagecontainingit.
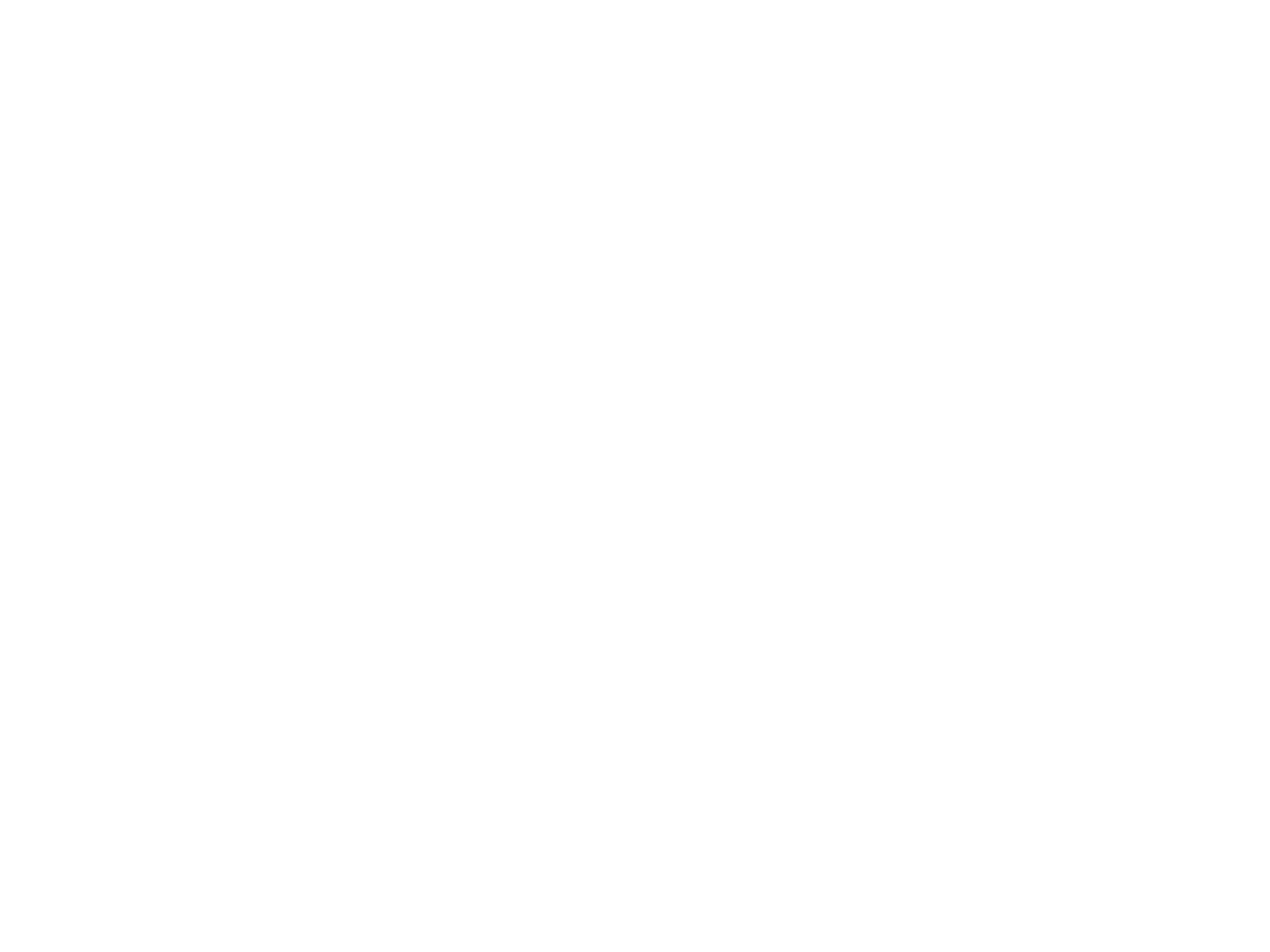
AccessControl
• public member(function/data)
– Canbecalled/modifiedfromoutside.
• protected
– Canbecalled/modifiedfromderivedclasses
• private
– Canbecalled/modifiedonlyfromthecurrentclass
• default ( if no access modifier stated )
– Usuallyreferredtoas“Friendly”.
– Canbecalled/modified/instantiatedfromthesamepackage.
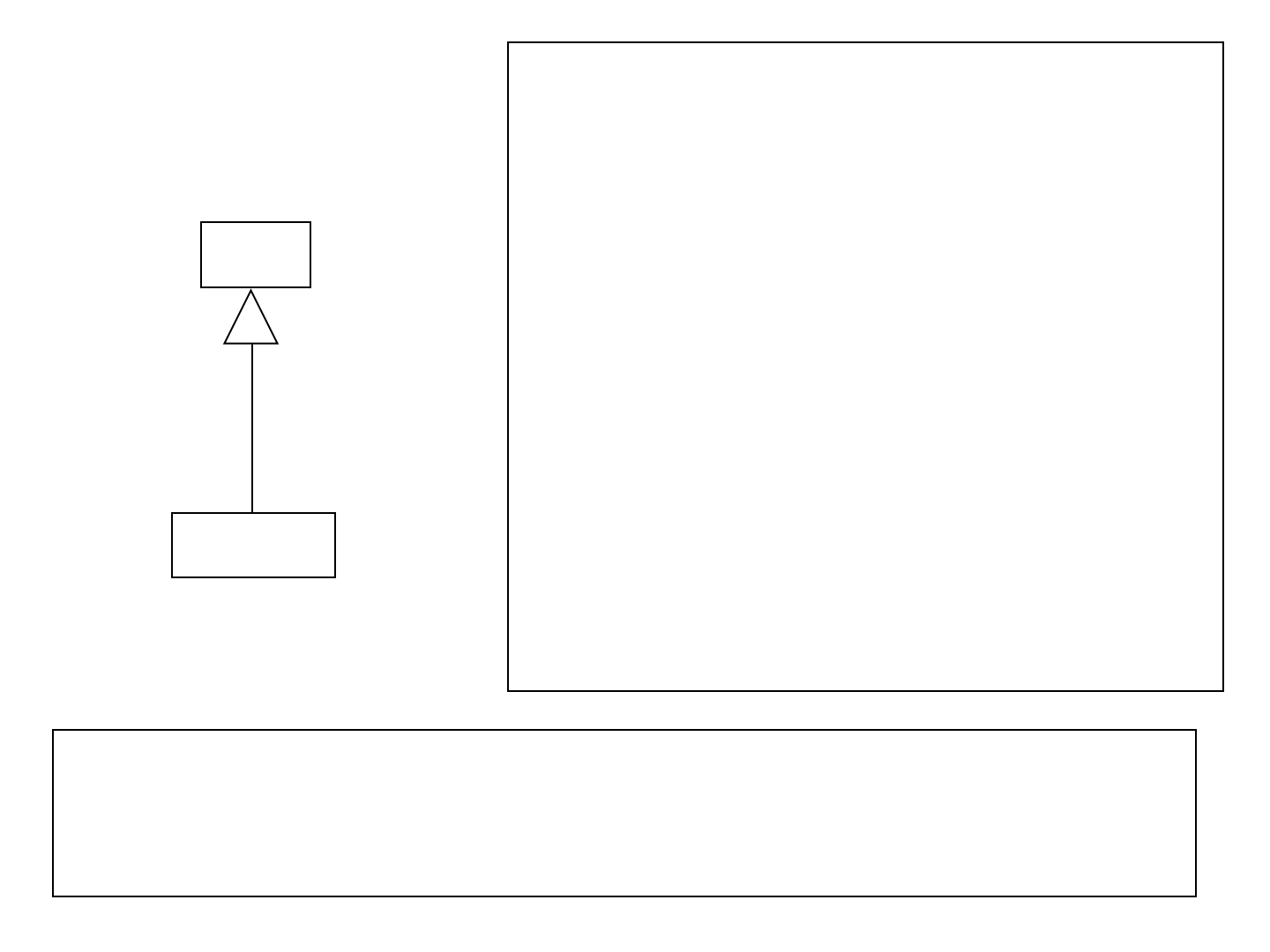
Inheritance
Base
Derived
class Base {
Base(){}
Base(int i) {}
protected void foo() {…}
}
class Derived extends Base {
Derived() {}
protected void foo() {…}
Derived(int i) {
super(i);
…
super.foo();
}
}
AsopposedtoC++,itispossibletoinheritonlyfromONEclass.
Pros avoidsmanypotentialproblemsandbugs.
Cons mightcausecodereplication
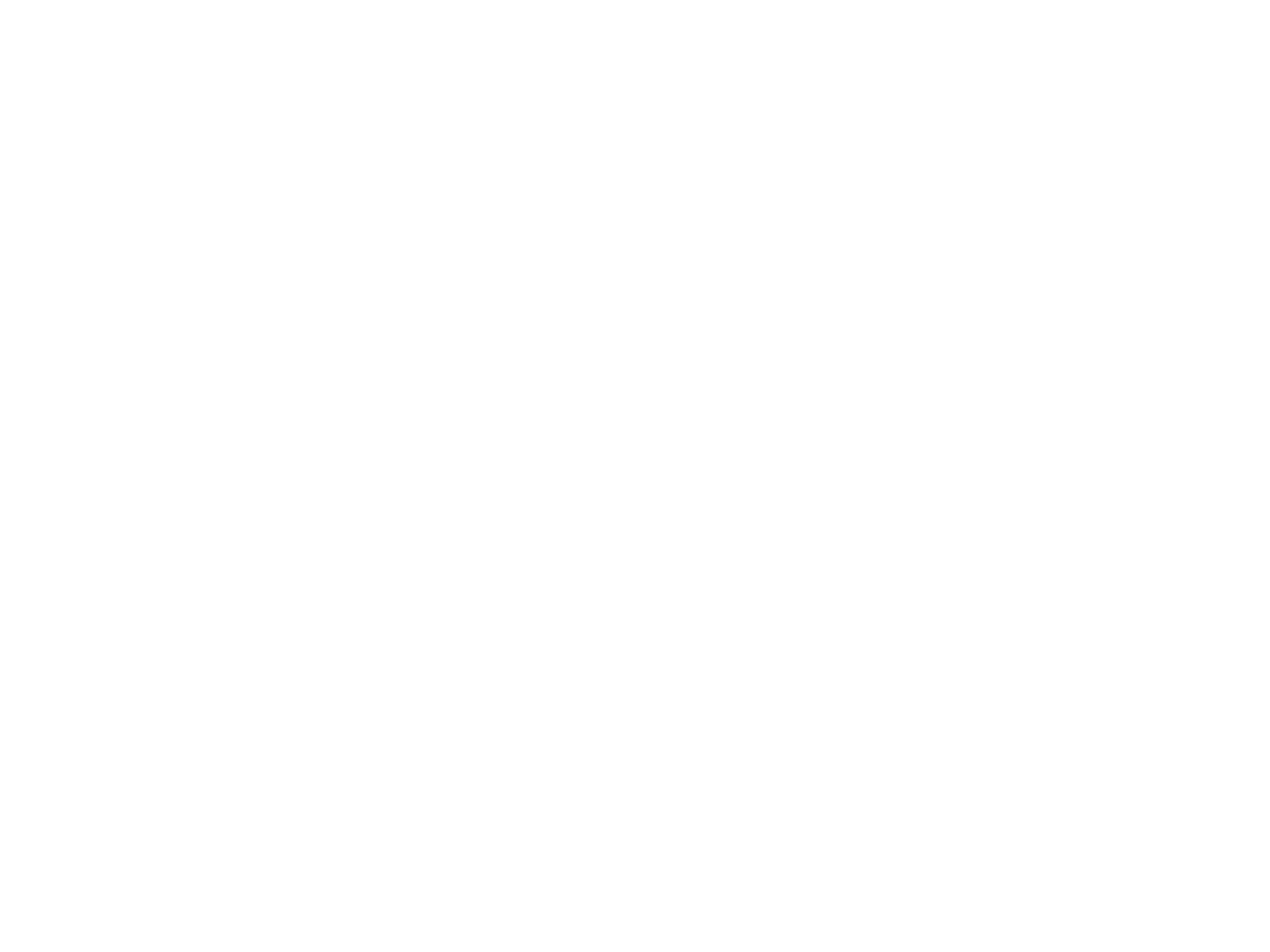
Polymorphism
• Inheritancecreatesan“isa”relation:
Forexample,ifBinheritsfromA,thanwesaythat
“BisalsoanA”.
Implicationsare:
– accessrights(Javaforbidsreducingaccessrights)-
derivedclasscanreceiveallthemessagesthatthebase
classcan.
– behavior
– preconditionandpostcondition
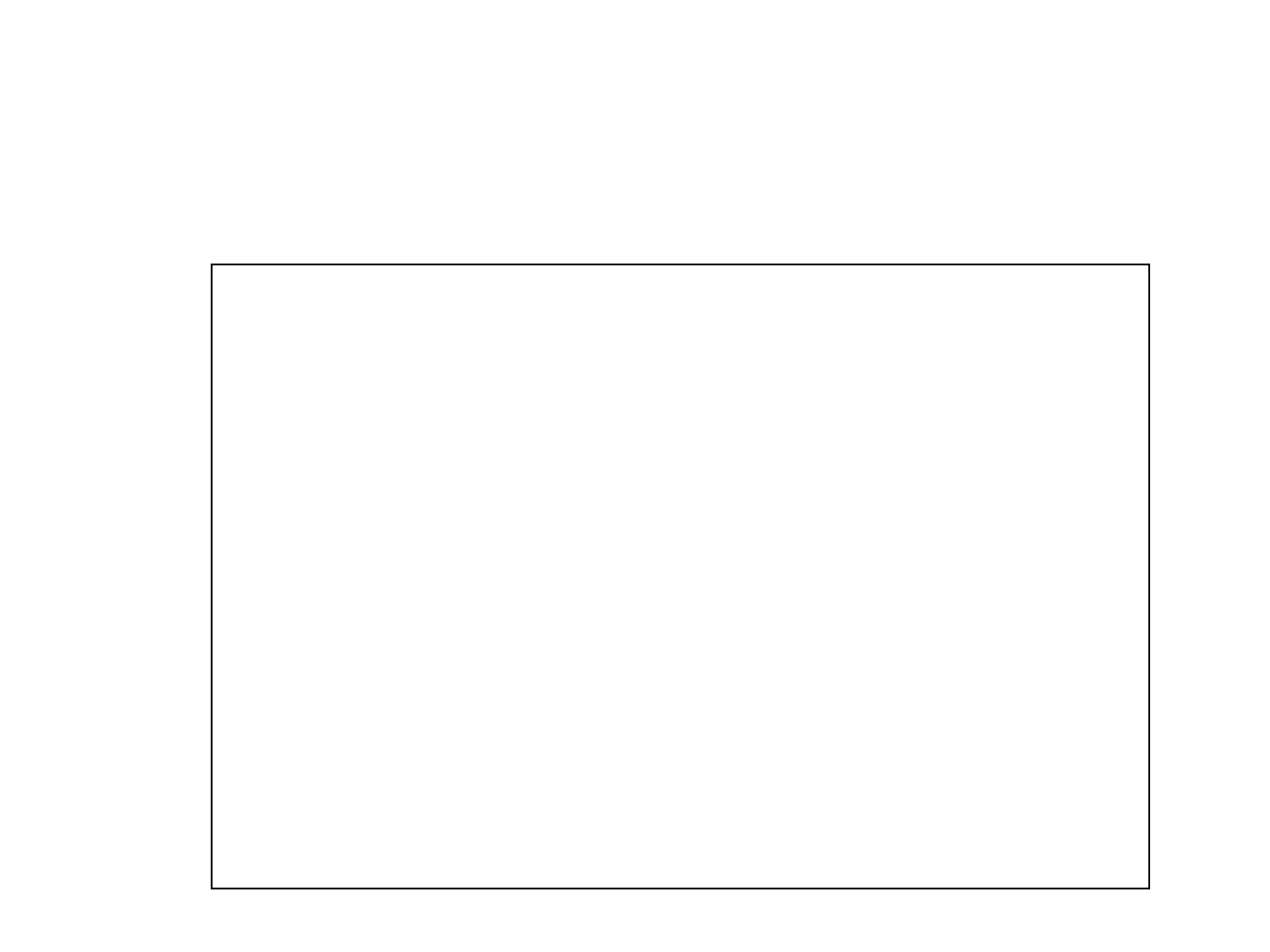
Inheritance(2)
• InJava,allmethodsarevirtual:
class Base {
void foo() {
System.out.println(“Base”);
}
}
class Derived extends Base {
void foo() {
System.out.println(“Derived”);
}
}
public class Test {
public static void main(String[] args) {
Base b = new Derived();
b.foo(); // Derived.foo() will be activated
}
}
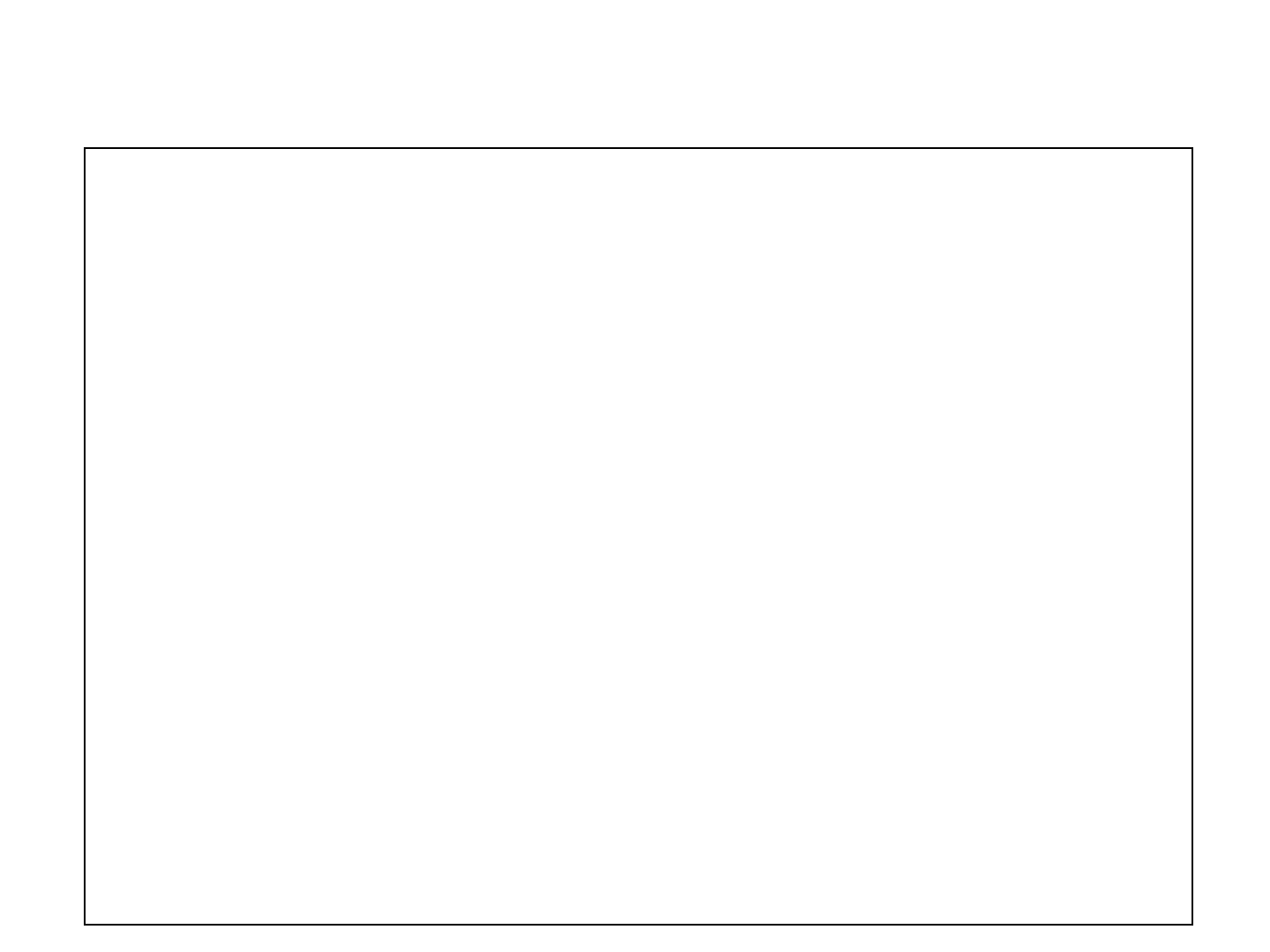
Inheritance(3)-Optional
class classC extends classB {
classC(int arg1, int arg2){
this(arg1);
System.out.println("In classC(int arg1, int arg2)");
}
classC(int arg1){
super(arg1);
System.out.println("In classC(int arg1)");
}
}
class classB extends classA {
classB(int arg1){
super(arg1);
System.out.println("In classB(int arg1)");
}
classB(){
System.out.println("In classB()");
}
}
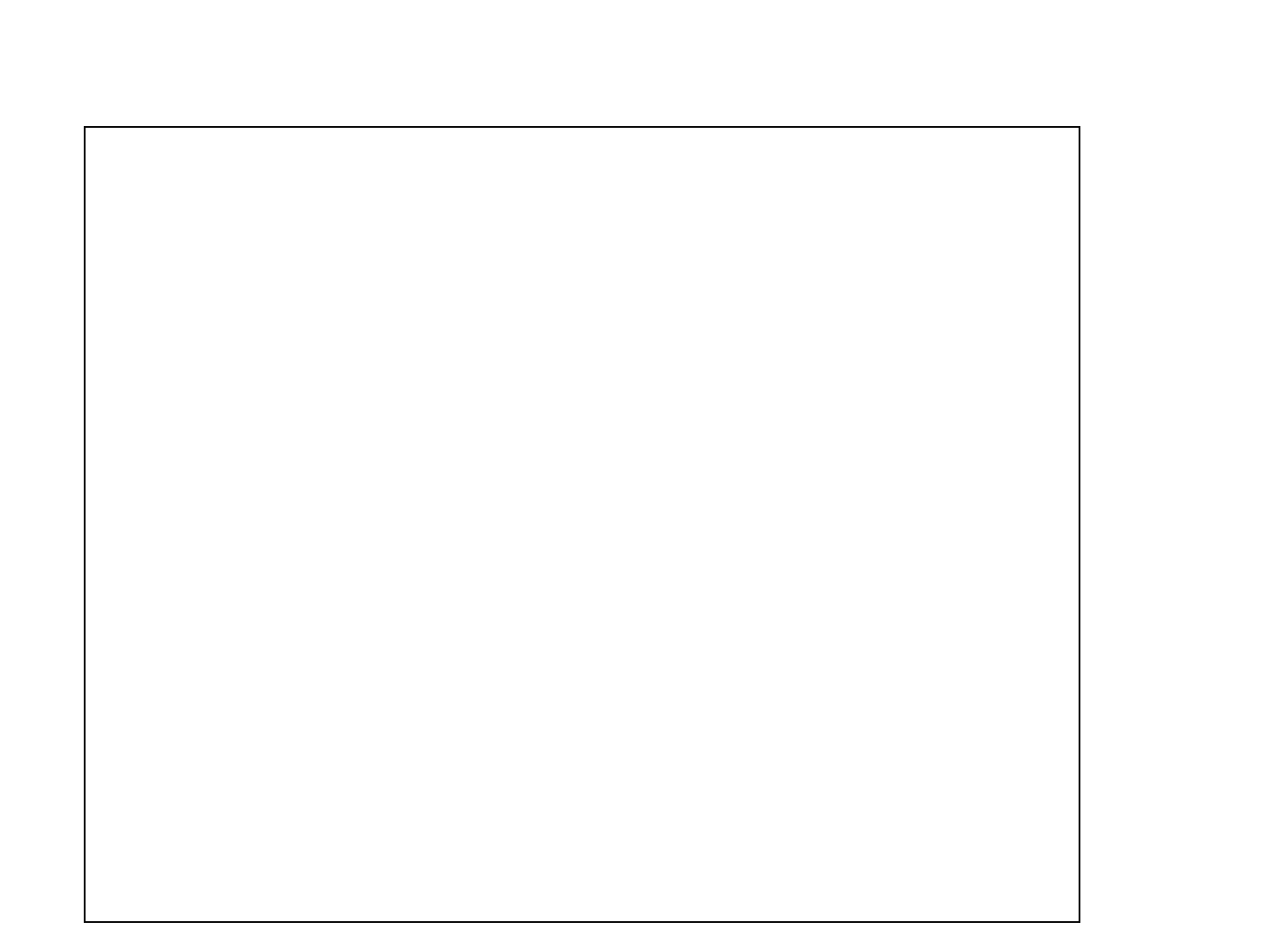
Inheritance(3)-Optional
class classA {
classA(int arg1){
System.out.println("In classA(int arg1)");
}
classA(){
System.out.println("In classA()");
}
}
class classB extends classA {
classB(int arg1, int arg2){
this(arg1);
System.out.println("In classB(int arg1, int arg2)");
}
classB(int arg1){
super(arg1);
System.out.println("In classB(int arg1)");
}
class B() {
System.out.println("In classB()");
}
}
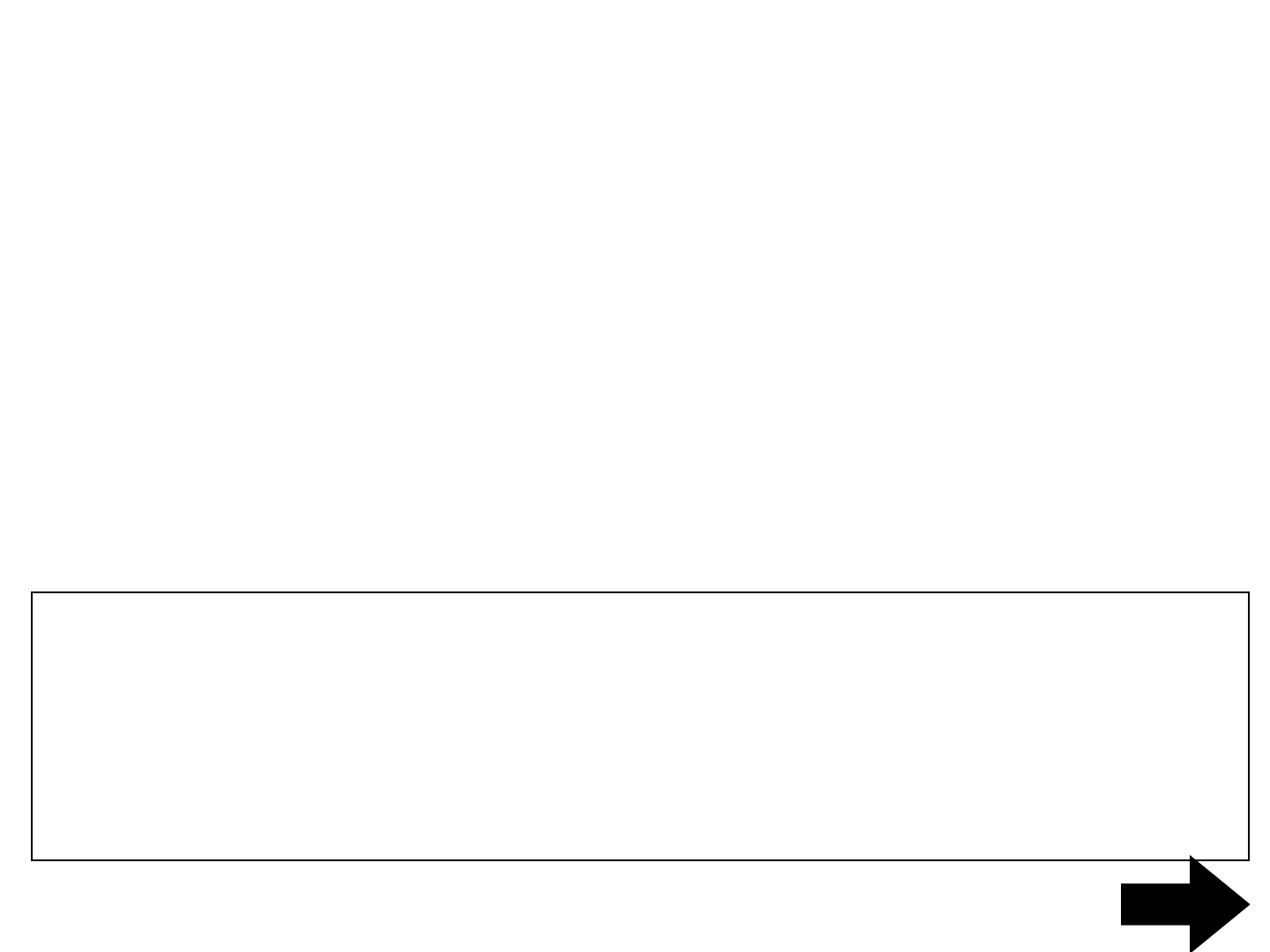
Abstract
• abstractmemberfunction,meansthatthefunctiondoes
nothaveanimplementation.
• abstractclass,isclassthatcannotbeinstantiated.
AbstractTest.java:6: class AbstractTest is an abstract class.
It can't be instantiated.
new AbstractTest();
^
1 error
NOTE:
Anabstractclassisnotrequiredtohaveanabstractmethodinit.
Butanyclassthathasanabstractmethodinitorthatdoes
notprovideanimplementationforanyabstractmethodsdeclared
initssuperclassesmustbedeclaredasanabstractclass.
Example
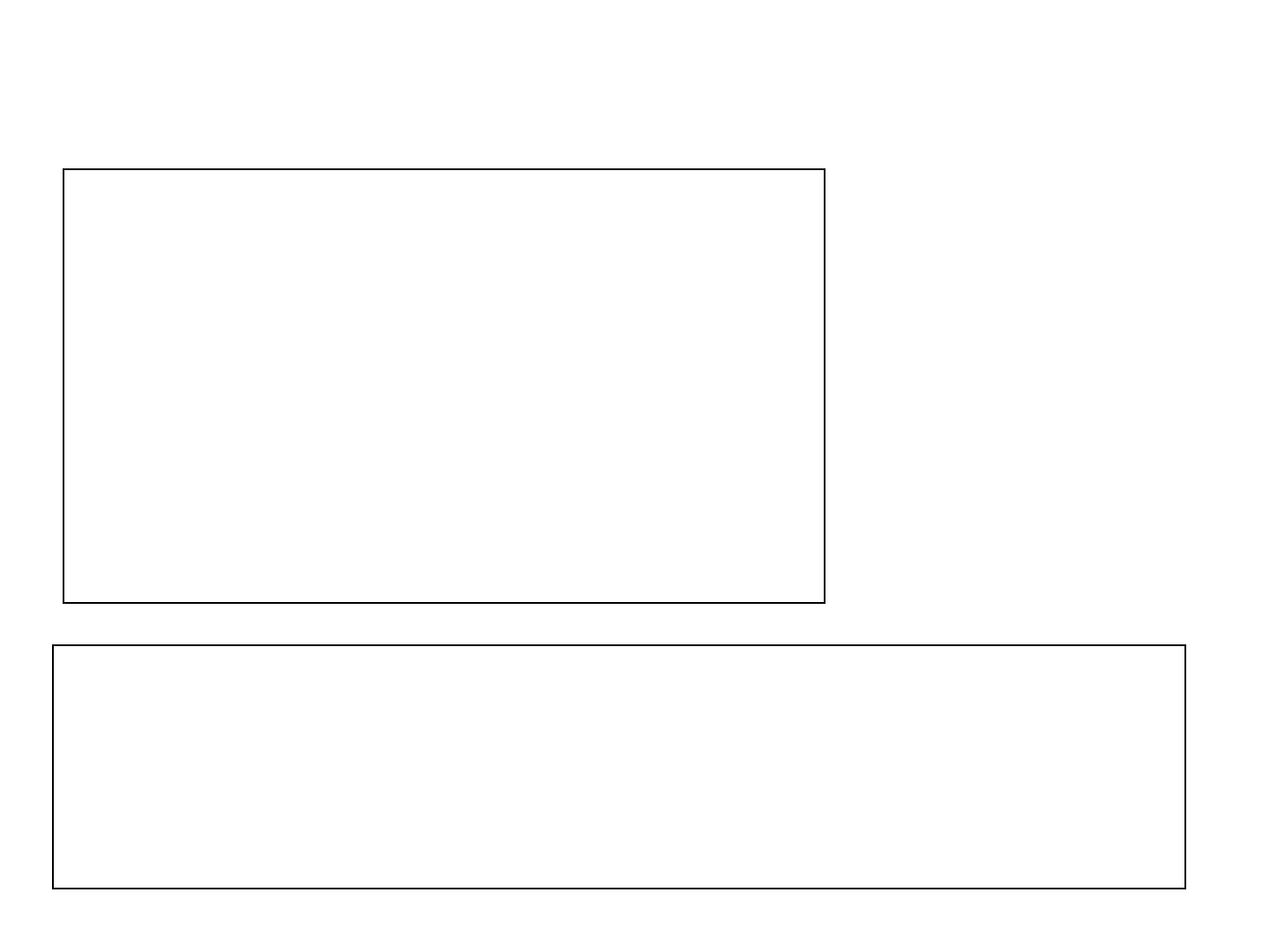
Abstract-Example
package java.lang;
public abstract class Shape {
public abstract void draw();
public void move(int x, int y) {
setColor(BackGroundColor);
draw();
setCenter(x,y);
setColor(ForeGroundColor);
draw();
}
}
package java.lang;
public class Circle extends Shape {
public void draw() {
// draw the circle ...
}
}
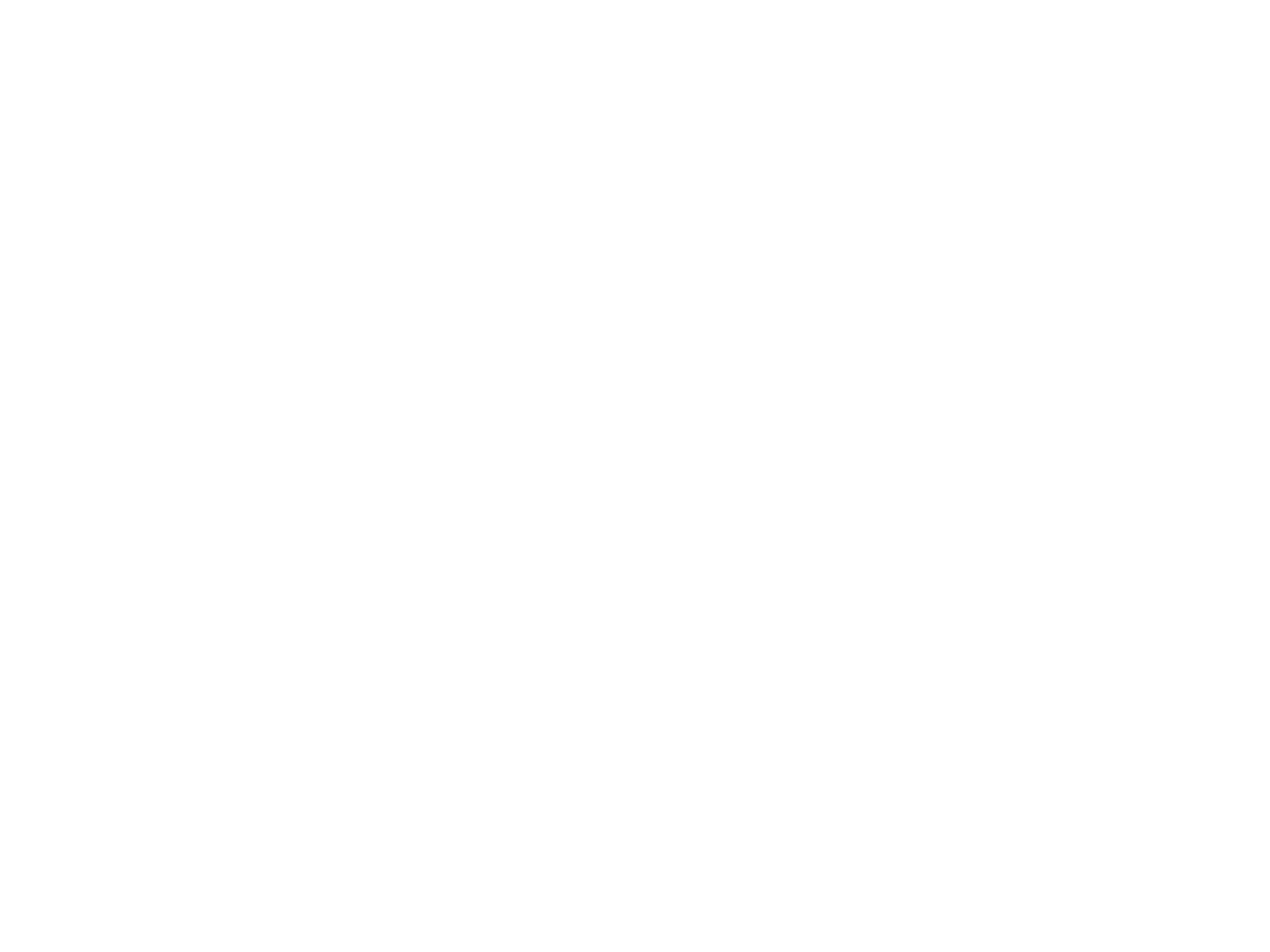
Interface
Interfacesareusefulforthefollowing:
· Capturingsimilaritiesamongunrelated
classeswithoutartificiallyforcingaclass
relationship.
· Declaringmethodsthatoneormoreclasses
areexpectedtoimplement.
· Revealinganobject'sprogramming
interfacewithoutrevealingitsclass.
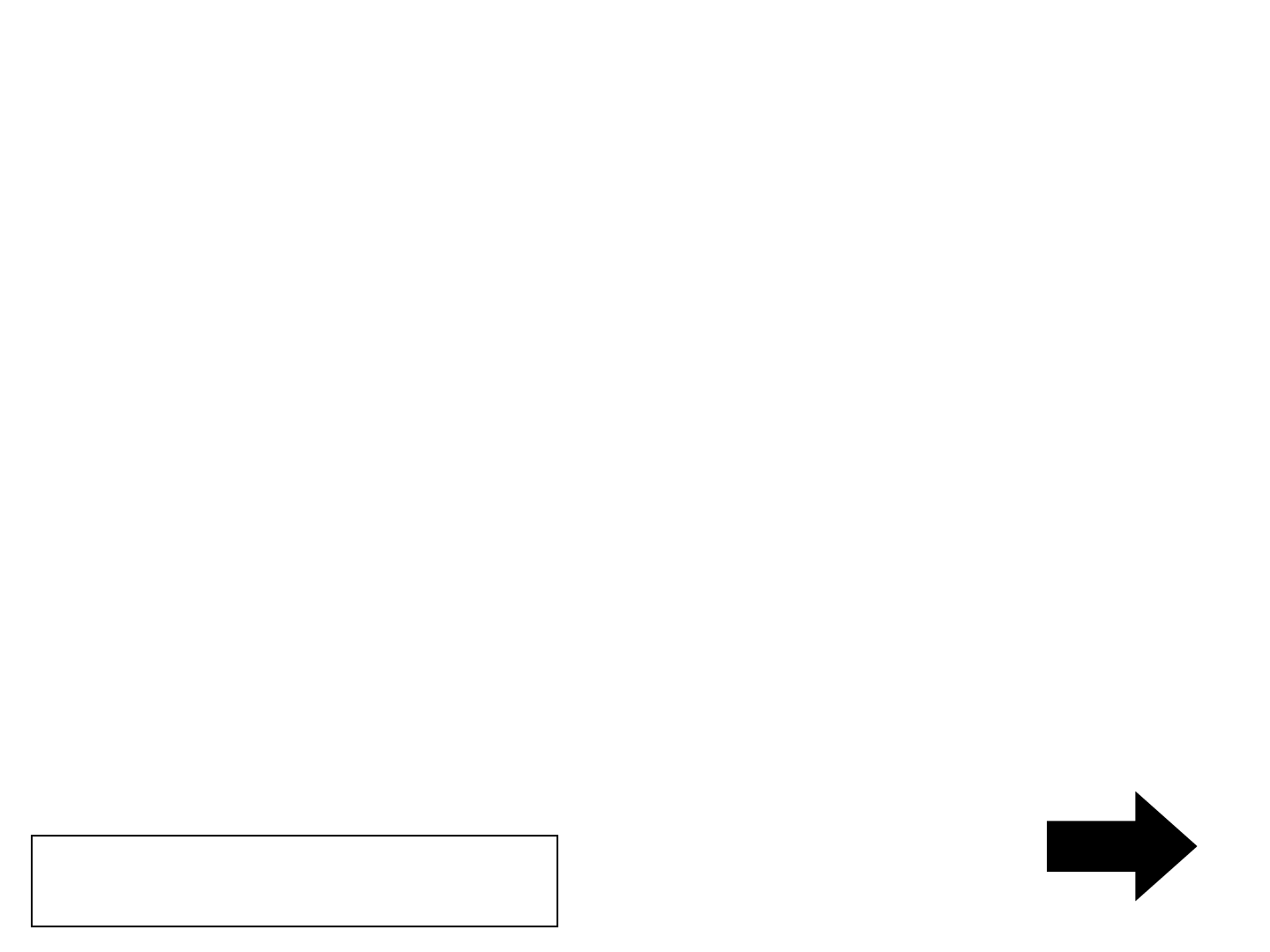
Interface
• abstract“class”
• Helpsdefininga“usagecontract”betweenclasses
• Allmethodsarepublic
• Java’scompensationforremovingthemultiple
inheritance.Youcan“inherit”asmanyinterfaces
asyouwant.
Example
-
*
Thecorrecttermis“toimplement”
aninterface
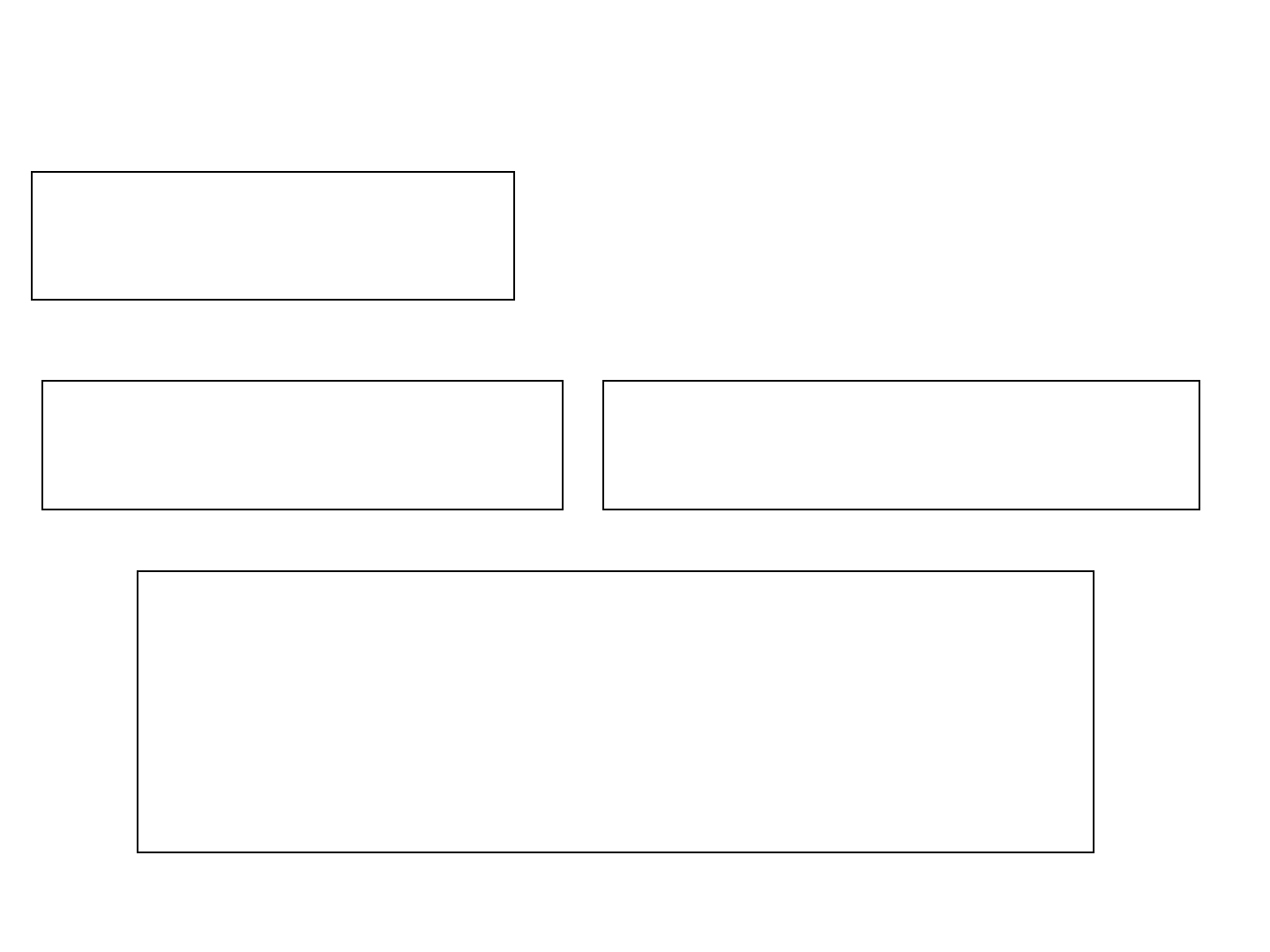
Interface
interface SouthParkCharacter {
void curse();
}
interface IChef {
void cook(Food food);
}
interface BabyKicker {
void kickTheBaby(Baby);
}
class Chef implements IChef, SouthParkCharacter {
// overridden methods MUST be public
// can you tell why ?
public void curse() { … }
public void cook(Food f) { … }
}
*accessrights(Javaforbidsreducingofaccessrights)
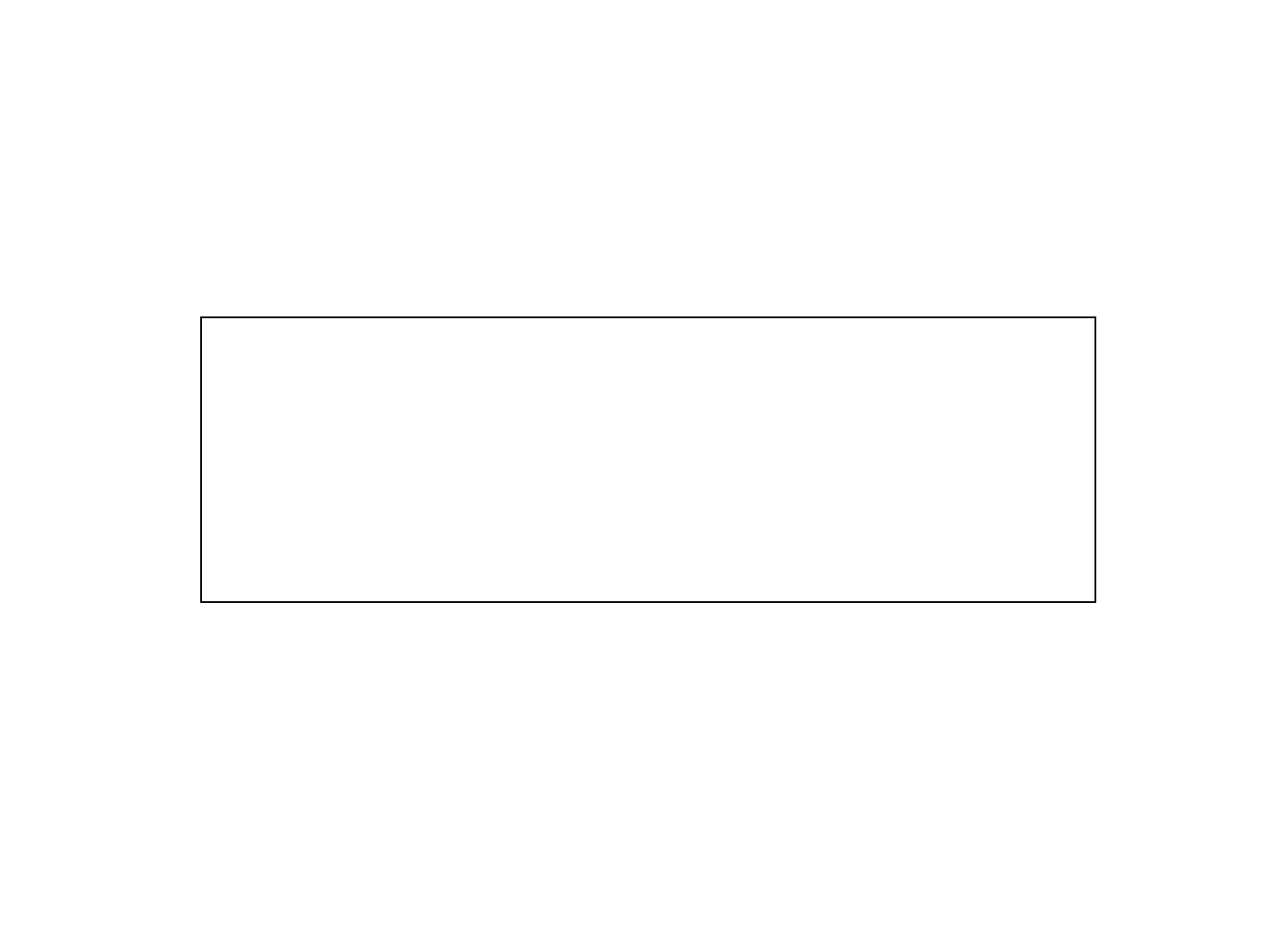
Whentouseaninterface?
Perfecttoolforencapsulatingthe
classesinnerstructure.Onlythe
interfacewillbeexposed
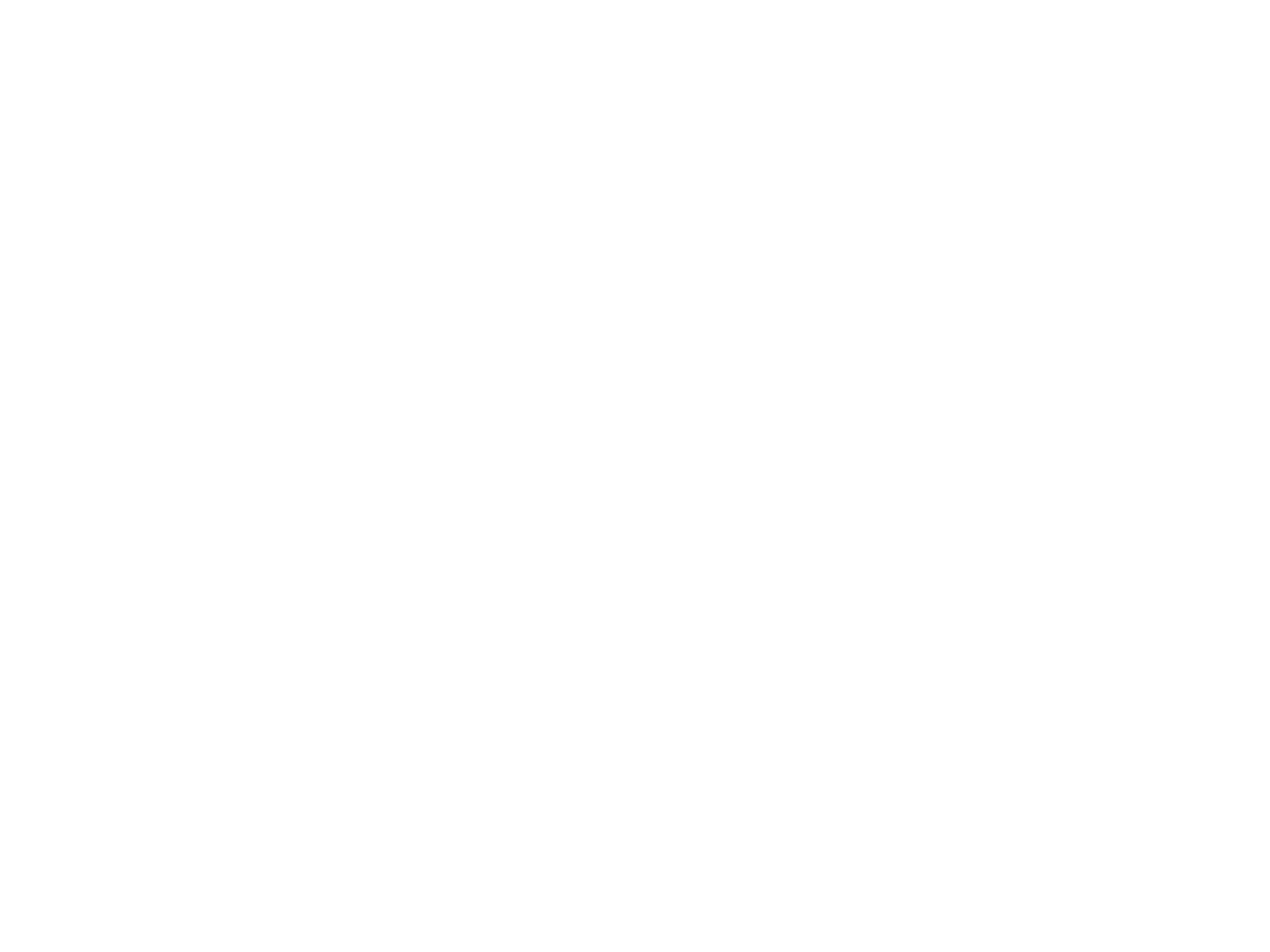
Collections
• Collection/container
– objectthatgroupsmultipleelements
– usedtostore,retrieve,manipulate,communicate
aggregatedata
• Iterator-objectusedfortraversingacollection
andselectivelyremoveelements
• Generics–implementationisparametricinthe
typeofelements
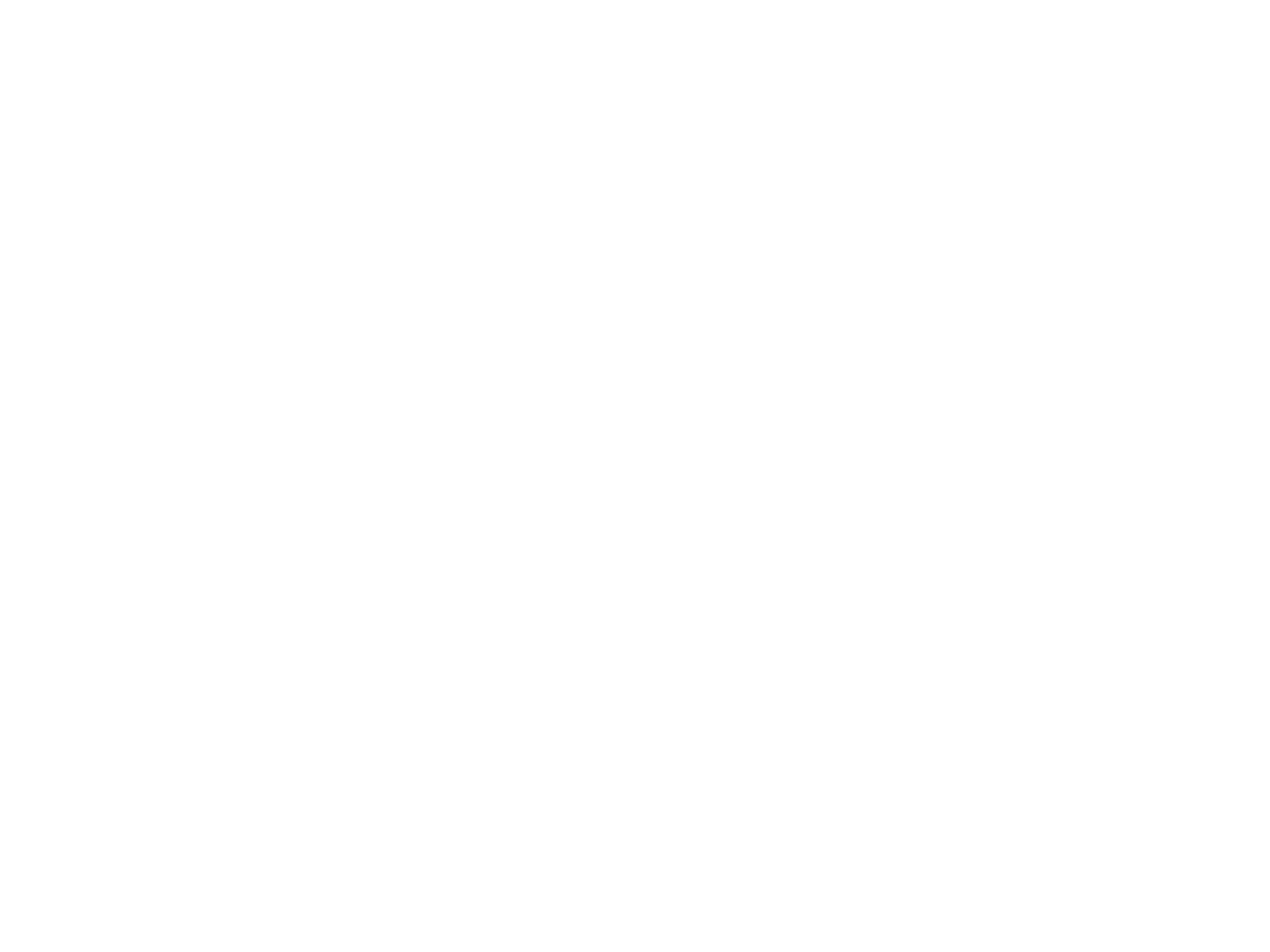
JavaCollectionFramework
• Goal:Implementreusabledata-structuresandfunctionality
• Collectioninterfaces-manipulatecollections
independentlyofrepresentationdetails
• Collectionimplementations-reusabledatastructures
List<String> list = new ArrayList<String>(c);
• Algorithms-reusablefunctionality
– computationsonobjectsthatimplementcollectioninterfaces
– e.g.,searching,sorting
– polymorphic:thesamemethodcanbeusedonmanydifferent
implementationsoftheappropriatecollectioninterface
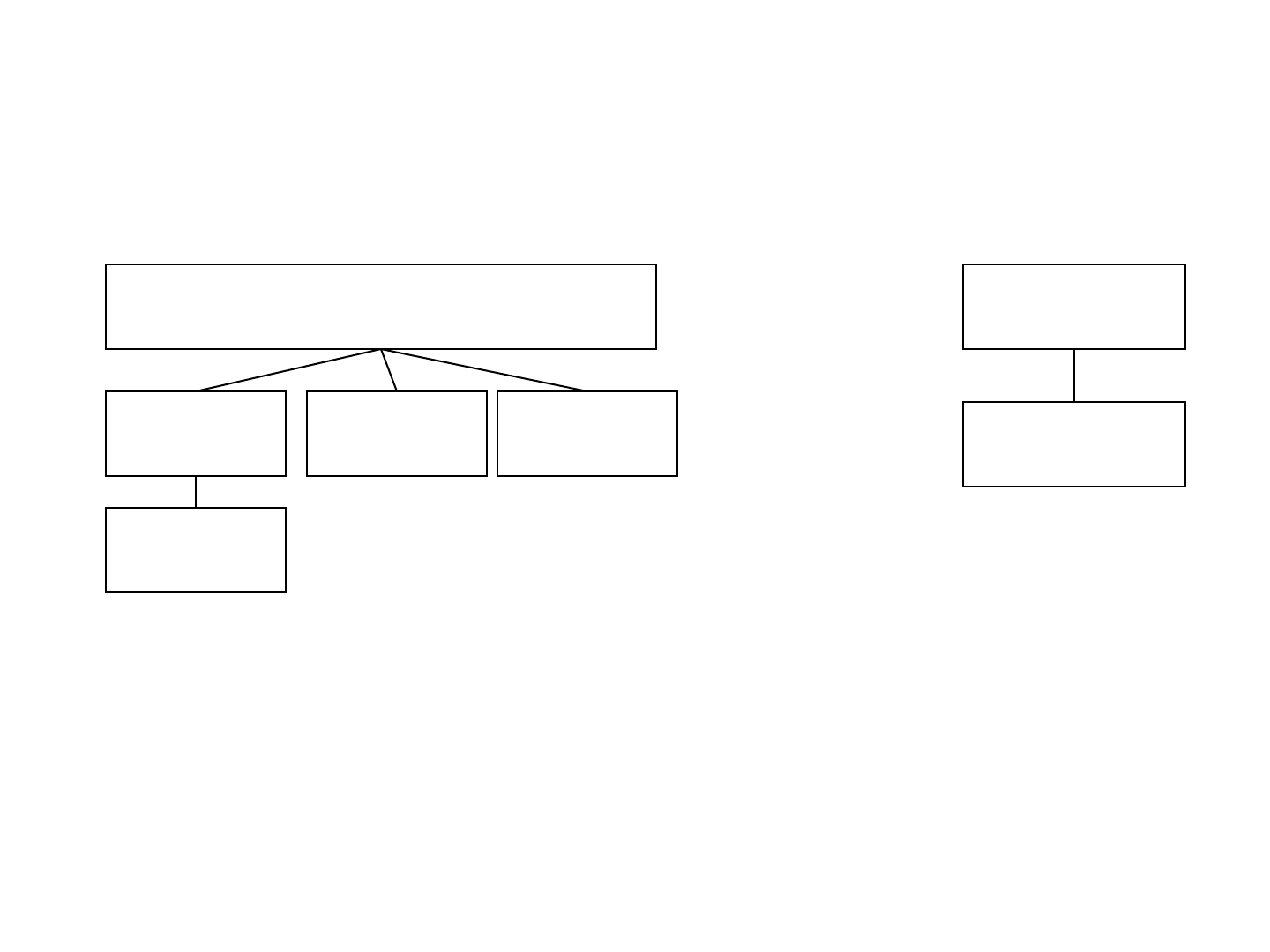
CollectionInterfaces
Collection
Set List Queue
SortedSet
Map
SortedMap
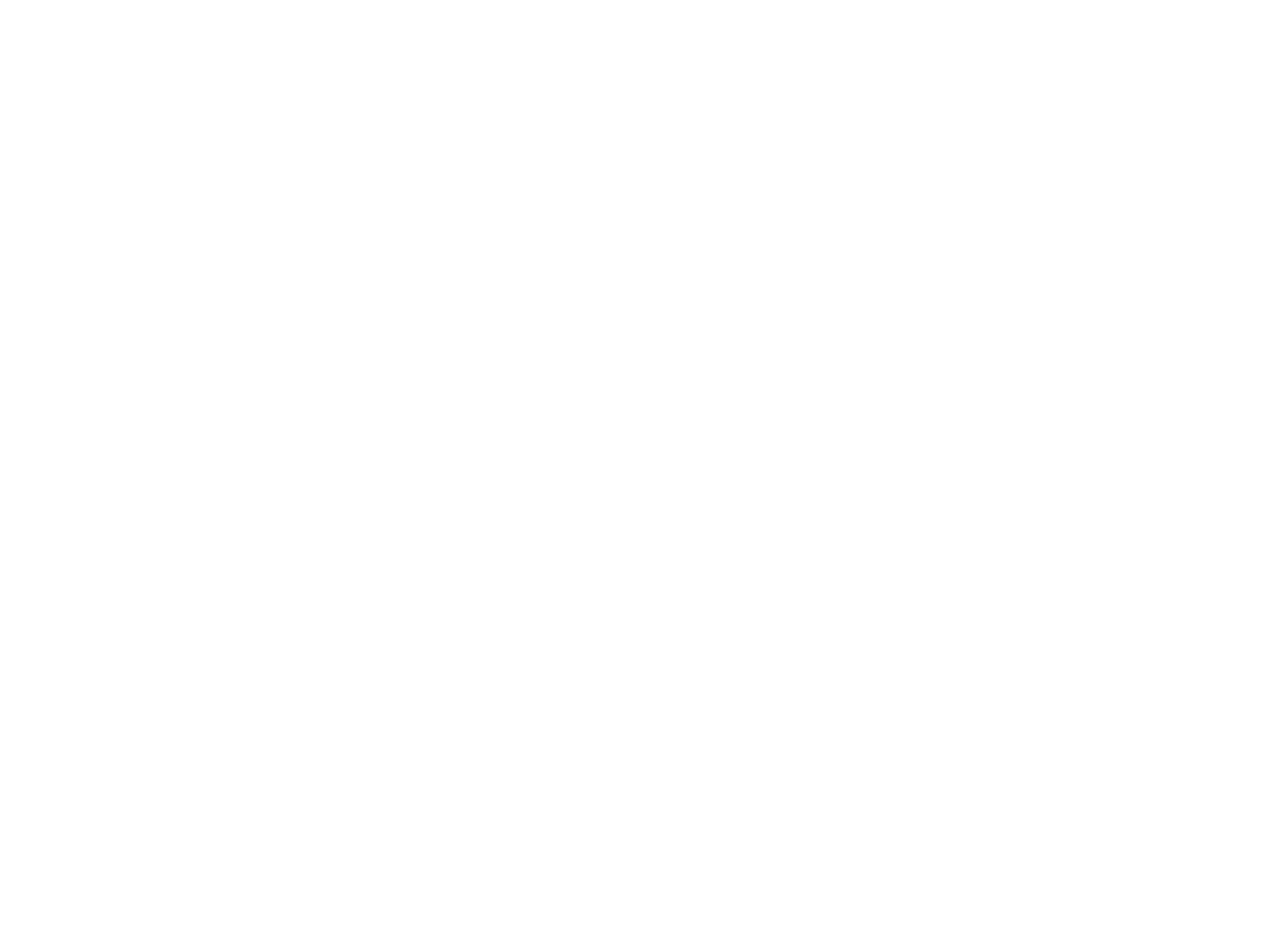
CollectionInterface
• BasicOperations
– intsize();
– booleanisEmpty();
– booleancontains(Objectelement);
– booleanadd(Eelement);
– booleanremove(Objectelement);
– Iteratoriterator();
• BulkOperations
– booleancontainsAll(Collection<?>c);
– booleanaddAll(Collection<?extendsE>c);
– booleanremoveAll(Collection<?>c);
– booleanretainAll(Collection<?>c);
– voidclear();
• ArrayOperations
– Object[]toArray();<T>T[]toArray(T[]a);}
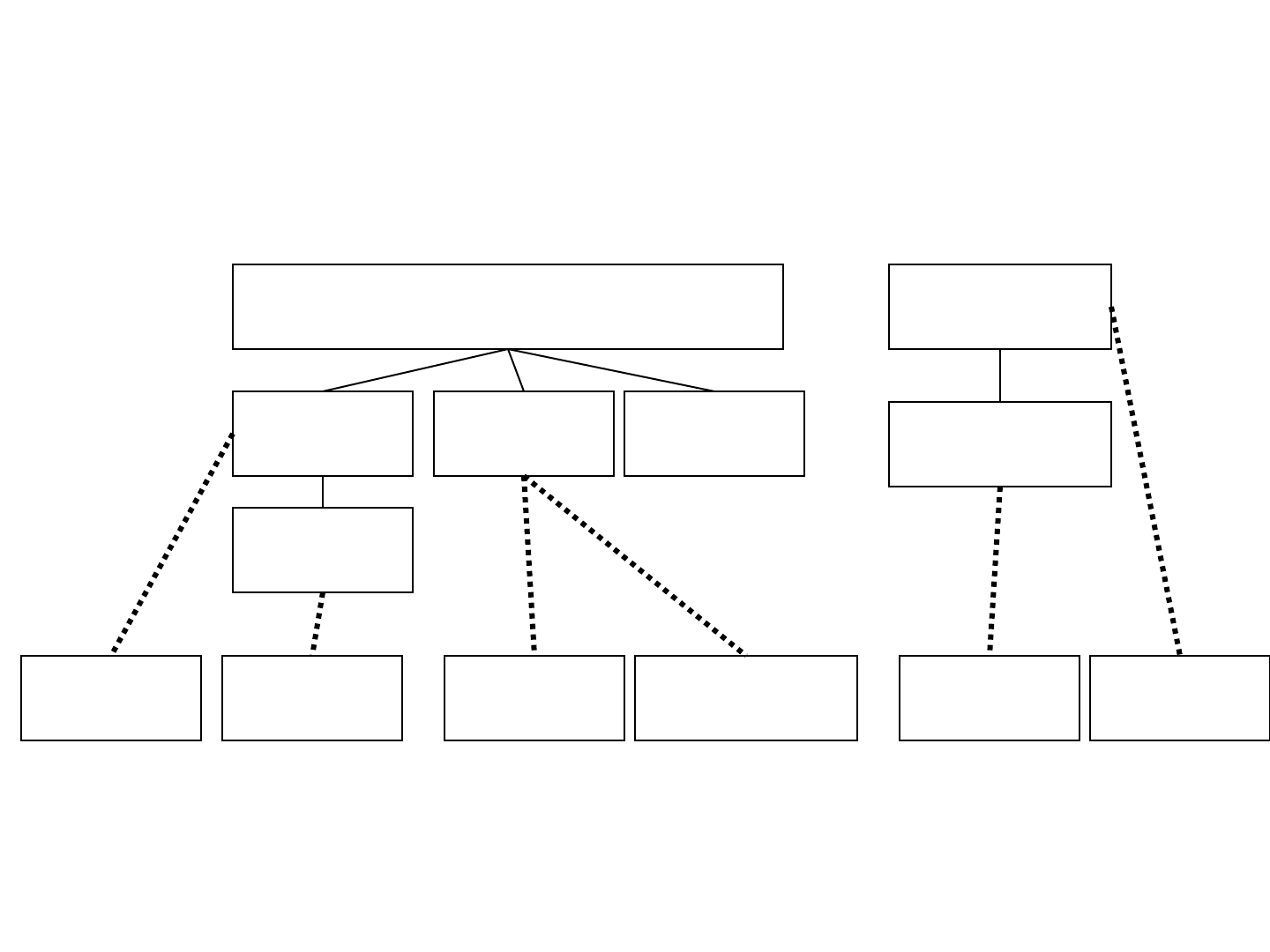
GeneralPurposeImplementations
Collection
Set List Queue
SortedSet
Map
SortedMap
HashSet HashMap
List<String>list1=newArrayList<String>(c);
ArrayListTreeSet TreeMapLinkedList
List<String>list2=newLinkedList<String>(c);
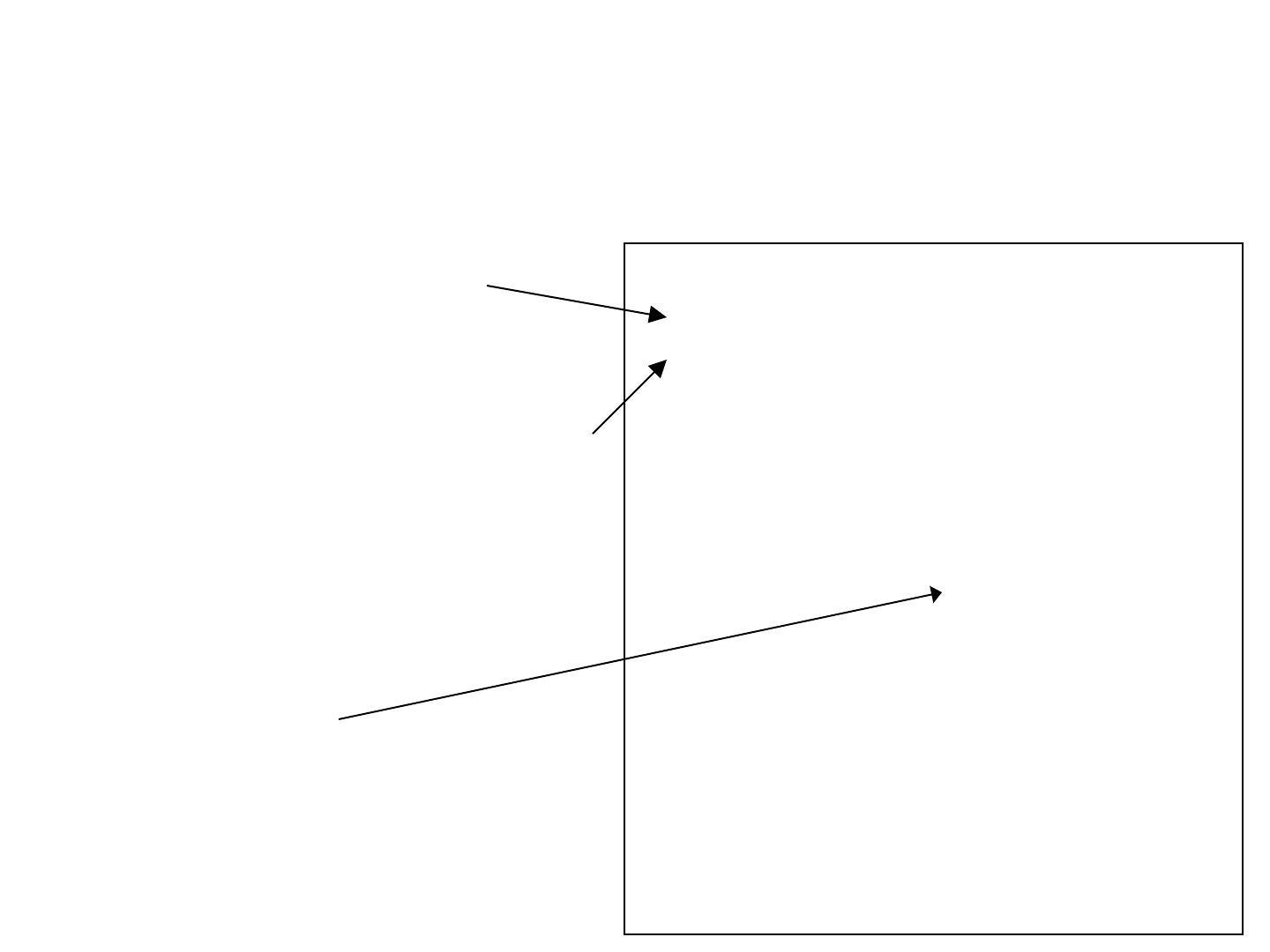
final
• final member data
Constantmember
• finalmember function
Themethodcan’tbe
overridden.
• final class
‘Base’isfinal,thusit
can’tbeextended
final class Base {
final int i=5;
final void foo() {
i=10;
//what will the compiler say
about this?
}
}
class Derived extends Base {
// Error
// another foo ...
void foo() {
}
}
(Stringclassisfinal)
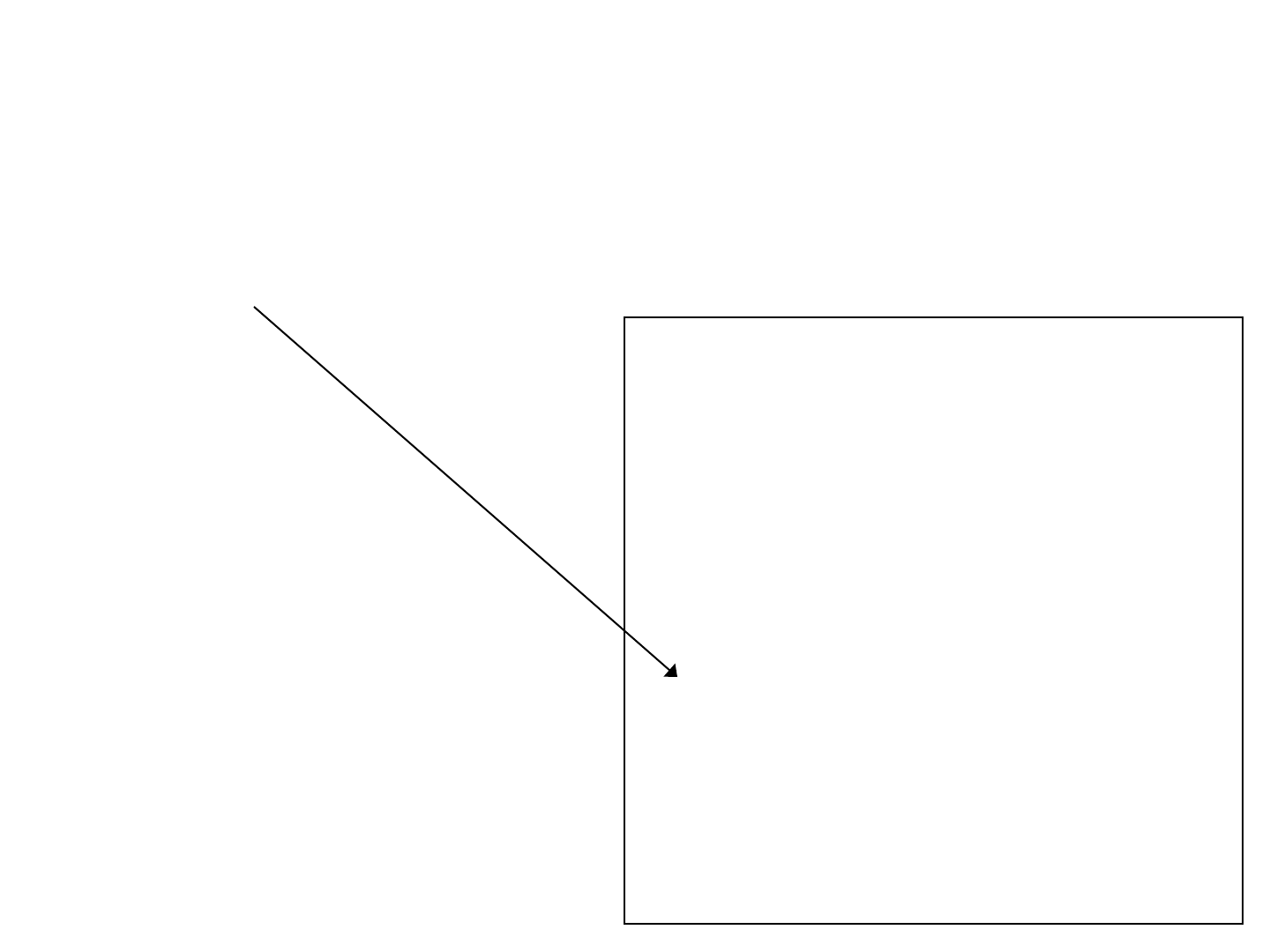
final
final class Base {
final int i=5;
final void foo() {
i=10;
}
}
class Derived extends Base {
// Error
// another foo ...
void foo() {
}
}
Derived.java:6: Can't subclass final classes: class Base
class class Derived extends Base {
^
1 error
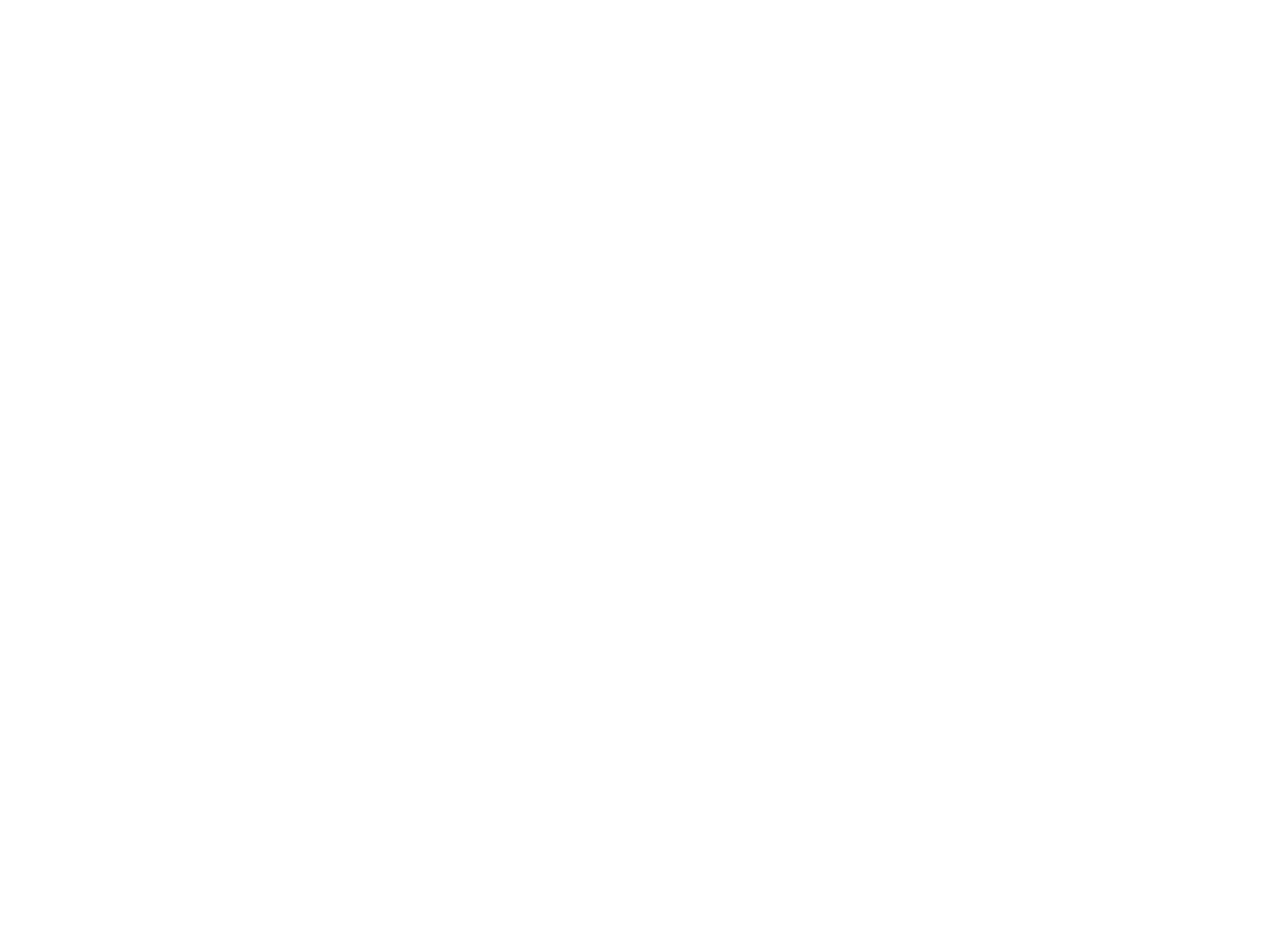
IO-Introduction
• Definition
– Streamisaflowofdata
• charactersreadfromafile
• byteswrittentothenetwork
• …
• Philosophy
– Allstreamsintheworldarebasicallythesame.
– Streamscanbedivided(asthename“IO”suggests)toInputand
Outputstreams.
• Implementation
– Incomingflowofdata(characters)implements“Reader”(InputStreamfor
bytes)
– Outgoingflowofdata(characters)implements“Writer”(OutputStreamfor
bytes–eg.Images,soundsetc.)
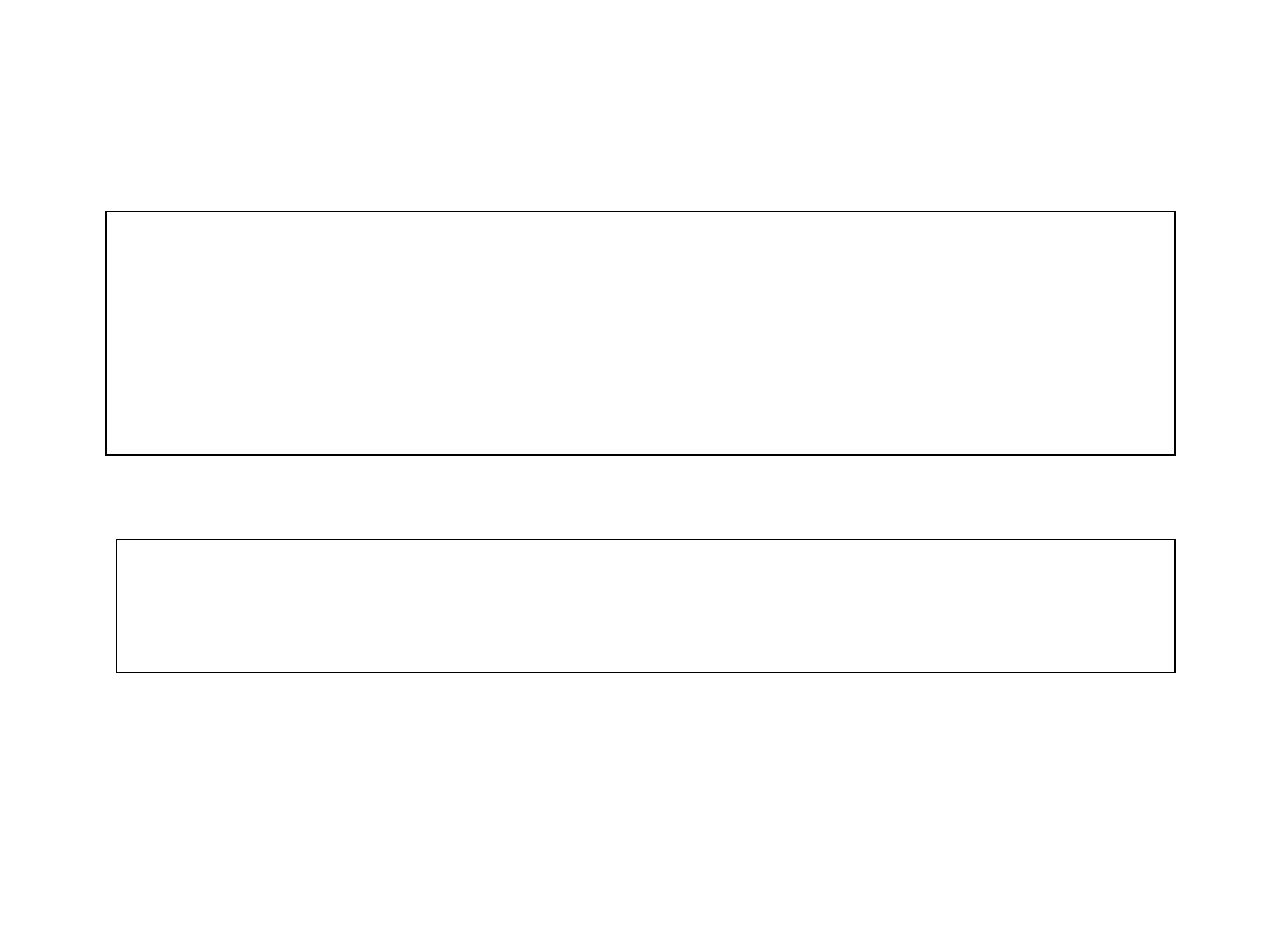
Exception-WhatisitandwhydoIcare?
Definition:Anexceptionisaneventthat
occursduringtheexecutionofaprogram
thatdisruptsthenormalflowofinstructions.
•ExceptionisanObject
•ExceptionclassmustbedescendentofThrowable.
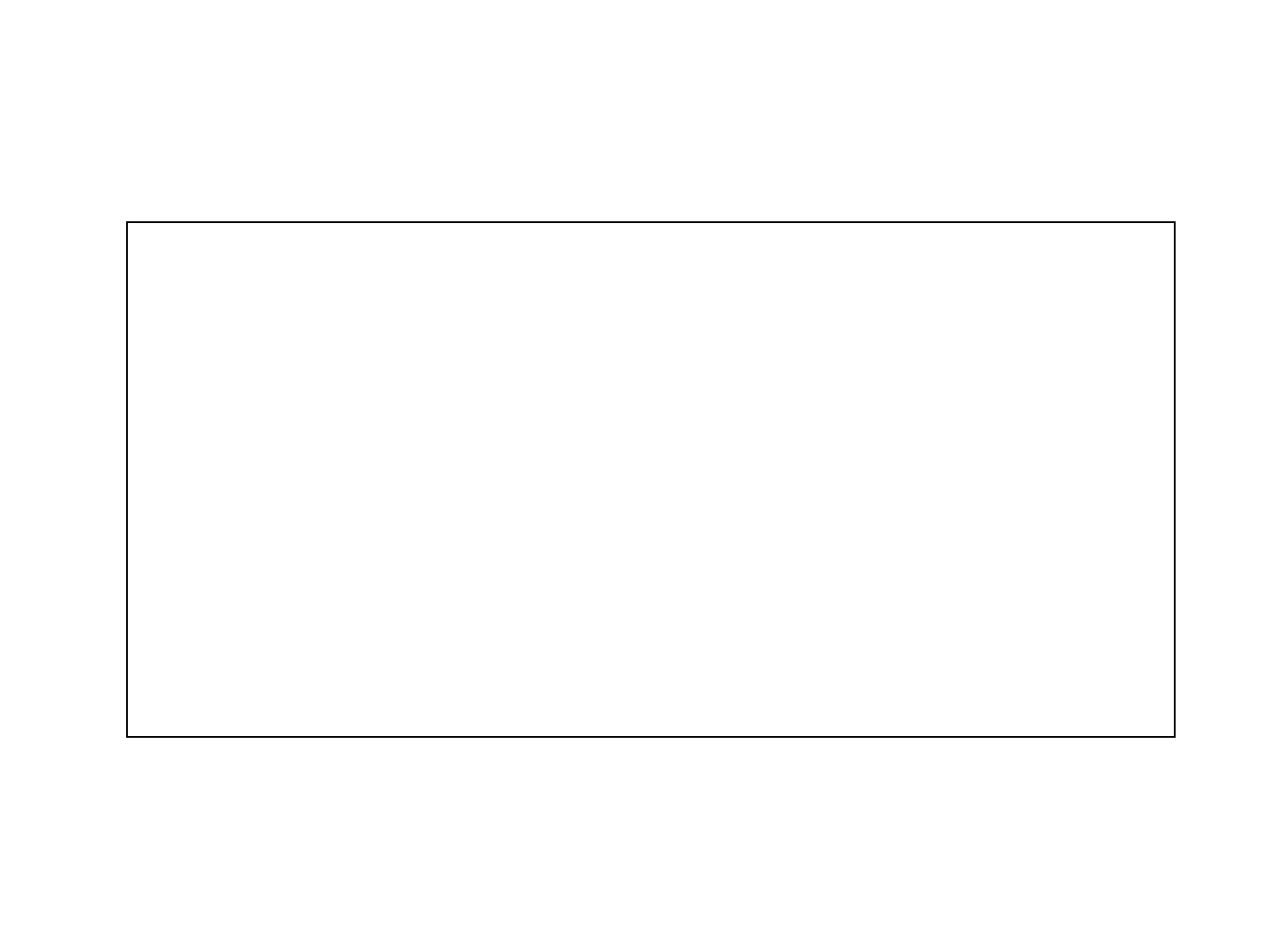
Exception-WhatisitandwhydoIcare?(2)
Byusingexceptionstomanageerrors,Java
programshavethefollowingadvantagesover
traditionalerrormanagementtechniques:
1:SeparatingErrorHandlingCodefrom"Regular"
Code
2:PropagatingErrorsUptheCallStack
3:GroupingErrorTypesandErrorDifferentiation
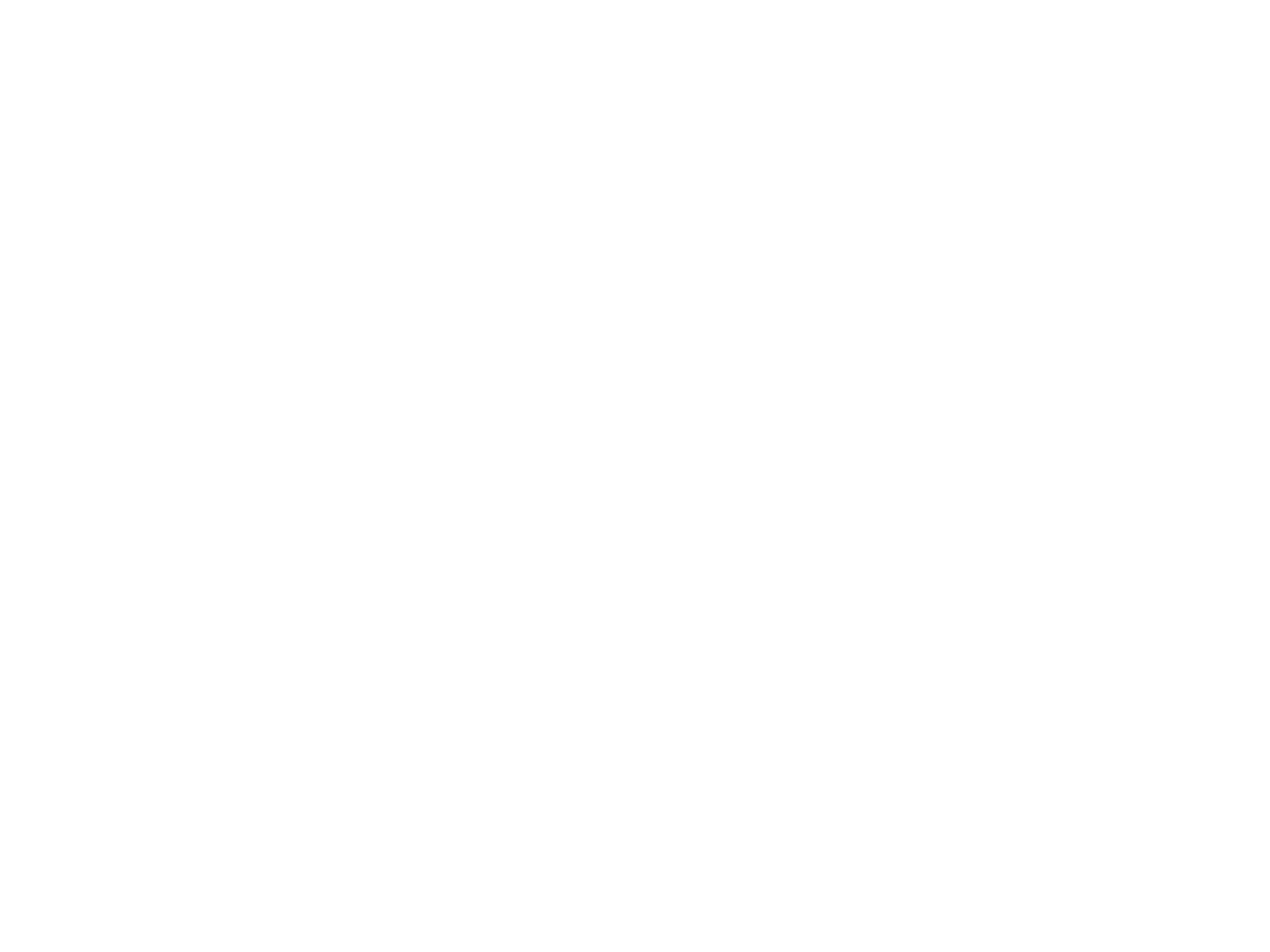
readFile {
open the file;
determine its size;
allocate that much memory;
read the file into memory;
close the file;
}
1 :Separating Error Handling Code from "Regular" Code (1)
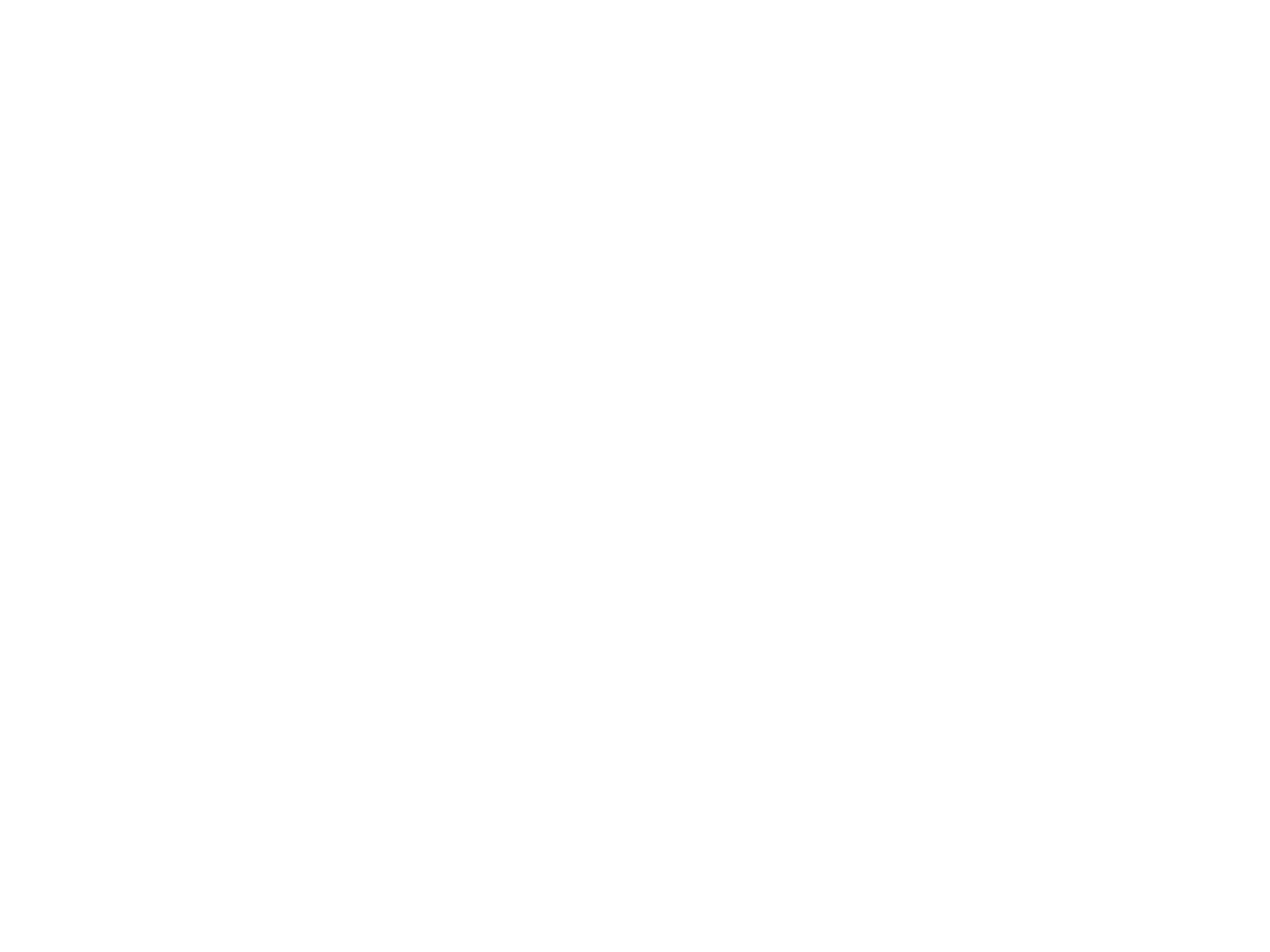
errorCodeType readFile {
initialize errorCode = 0;
open the file;
if (theFileIsOpen) {
determine the length of the file;
if (gotTheFileLength) {
allocate that much memory;
if (gotEnoughMemory) {
read the file into memory;
if (readFailed) {
errorCode = -1;
}
} else {
errorCode = -2;
}
} else {
errorCode = -3;
}
close the file;
if (theFileDidntClose && errorCode == 0) {
errorCode = -4;
} else {
errorCode = errorCode and -4;
}
} else {
errorCode = -5;
}
return errorCode;
}
1 :Separating Error Handling Code from "Regular" Code (2)
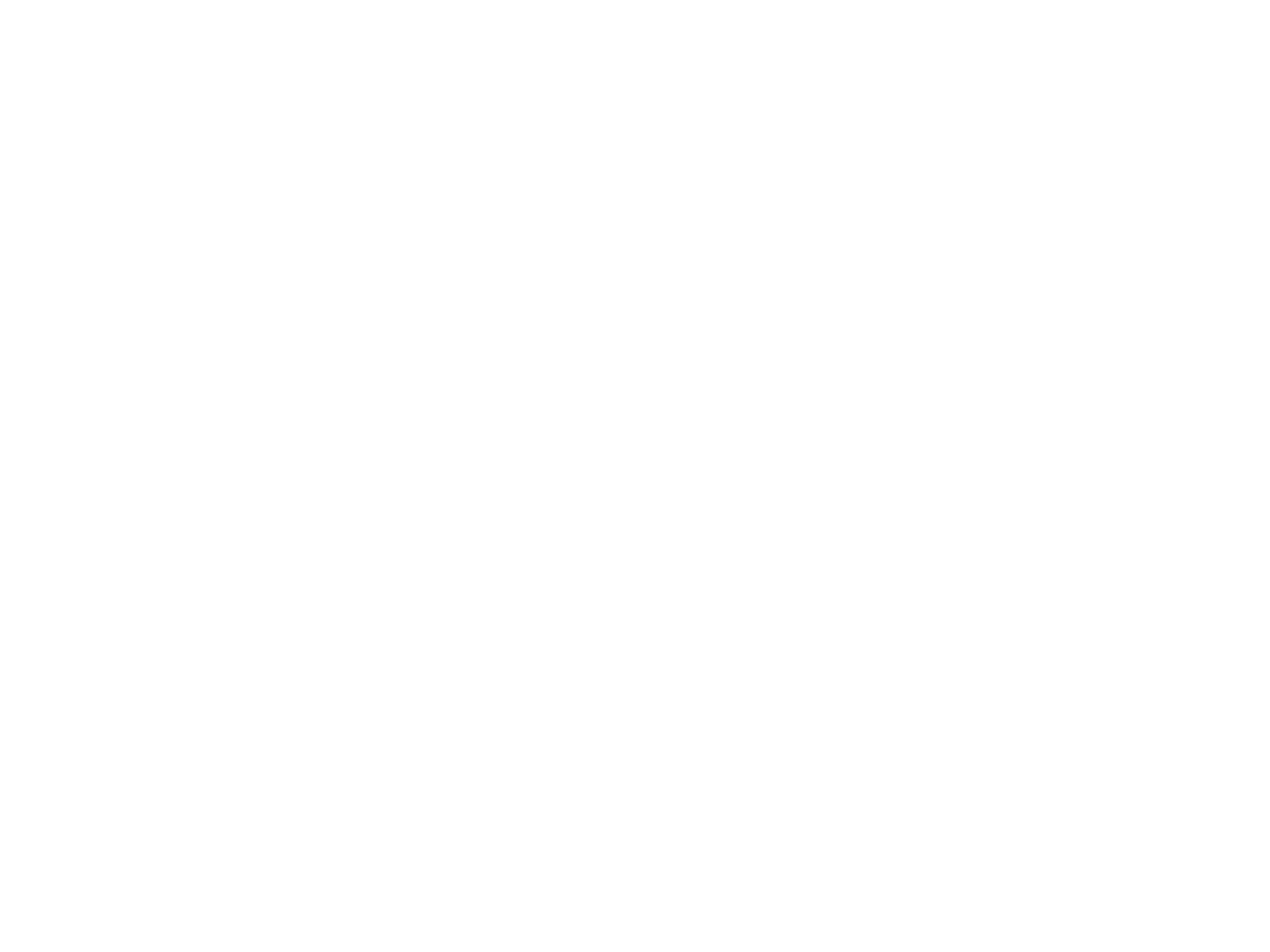
readFile {
try {
open the file;
determine its size;
allocate that much memory;
read the file into memory;
close the file;
} catch (fileOpenFailed) {
doSomething;
} catch (sizeDeterminationFailed) {
doSomething;
} catch (memoryAllocationFailed) {
doSomething;
} catch (readFailed) {
doSomething;
} catch (fileCloseFailed) {
doSomething;
}
}
1:SeparatingErrorHandlingCodefrom"Regular"Code (3)
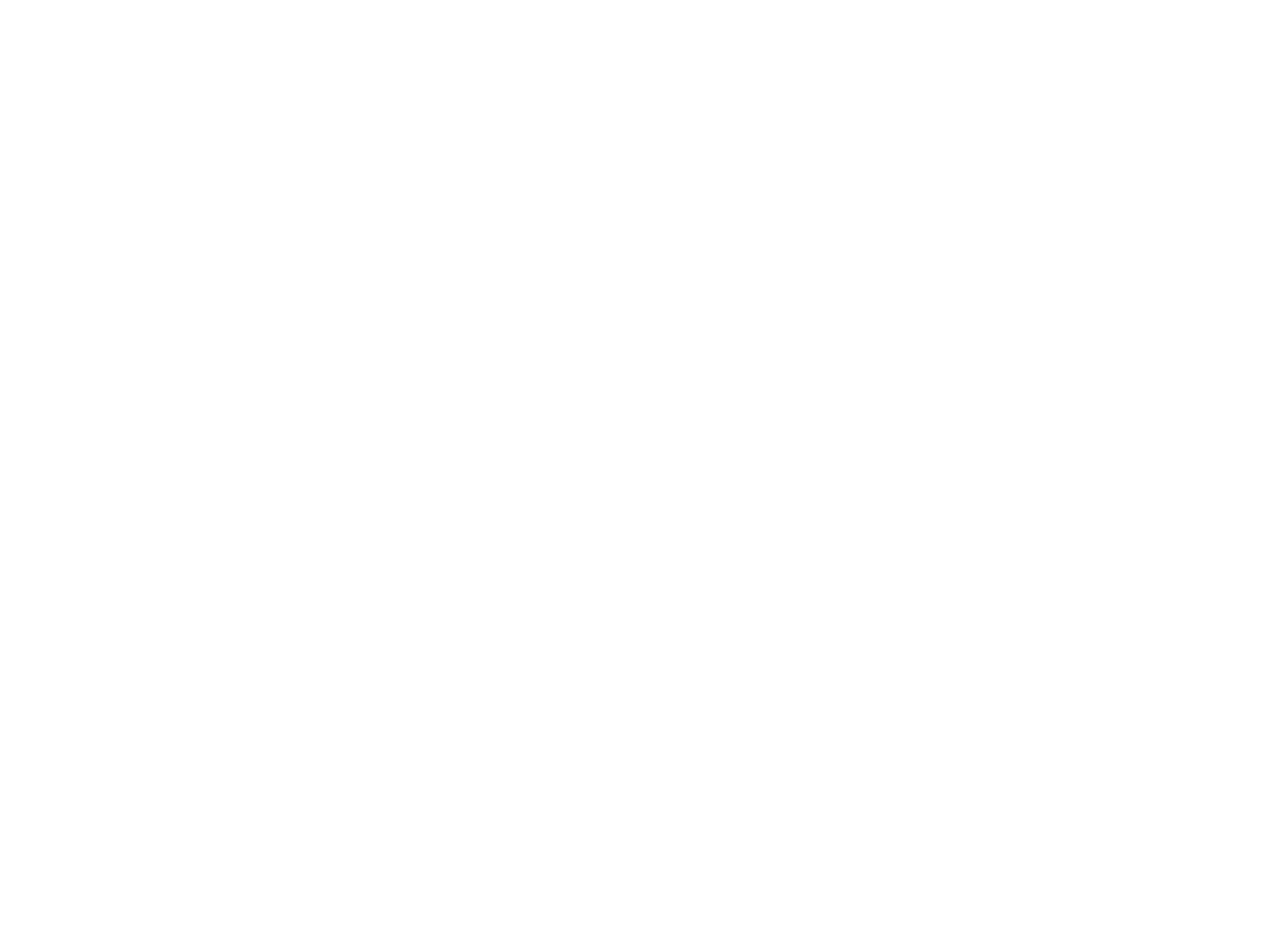
method1 {
try {
call method2;
} catch (exception) {
doErrorProcessing;
}
}
method2 throws exception {
call method3;
}
method3 throws exception {
call readFile;
}
2:PropagatingErrorsUptheCallStack